Visual C++
CloudLabs
Projects
Assignment
24x7 Support
Lifetime Access
.
Course Overview
This intensive course teaches C++ and Windows programming using Visual C++ and the Microsoft Foundation Class Library (MFC). The course consists of two modules. In the first module object oriented concepts are introduced. The Visual C++ development environment is demonstrated. The C++ class construct is introduced and its key features elaborated step-by-step, providing full implementation of abstract data types. C++ memory management is discussed. Function and operator overloading and the use of references are covered. The scope and access control mechanisms of C++ are described. Inheritance is introduced. The use of virtual functions to implement polymorphism is discussed
At the end of the training, participants will be able to:
Pre-requisite
A background of programming in another language such as C, Java, or C#.
Duarion
5 days
Course Outline
- Objects
- Information Hiding and Encapsulation
- Abstract Data Types
- Methods and Messages
- Classes
- Class Inheritance
- Polymorphism
- Data Encapsulation in C and C++
- Definition of C++ Classes
- Member Data and Functions this Pointer
- Abstract Data Types
- Organizing Code for Classes
- Function Prototypes and Type Checking
- Conversion of Parameters
- Default Arguments
- Inline Functions
- Function Overloading
- Constructors and Initializations
- Object Creation and Destruction
- Destructors
- Multiple Constructors in a Class
- Hidden Constructors
- Static, Automatic and Heap Memory
- New and Delete
- Handling Memory Allocation Errors
- Hiding Details of Memory Management in a Class
- Implementing a Dynamic String Class
- Call by Value
- Reference Declarations
- Reference Arguments
- Copy Constructor
- Constant Arguments and Functions
- Operator Overloading
- Semantics of Assignment
- Initialization vs. Assignment
- Overloading Assignment
- Type Conversions
- Scope in C++
- Friend Functions
- Const and Enumeration Types
- Static Members
- Inheritance for Modeling and Reuse
- Class Derivation
- Access Control
- Base Class Initialization
- Composition
- Initializing Class Type Members
- Virtual Functions and Dynamic Binding
- Polymorphism in C++
- Pointer Conversion
- Virtual Destructors
- Abstract Classes and Pure Virtual Functions
- Visual C++ Development Environment
- Visual Studio
- Managing Projects
- Compiling and Linking
- Debugging
- Structure of Windows Programs
- Application Frameworks
- Class Hierarchy of the MFC Library
- CWinApp and CFrameWnd Classes
- Event-Driven Programming
- Windows Messages
- Message Maps
- Mouse
- Using ClassWizard
- Using Spy++
- Invalidating the Client Area
- Keyboard
- Device Contexts
- GDI Object Creation and Cleanup
- Colors
- Pens and Brushes
- Drawing with Text
- MFC Encapsulation of Windows
- Window Creation and Destruction
- Focus and Activation
- Sending and Posting Messages
- Overlapped, Popup and Child Windows
- Child Windows and Owned Windows
- Using AppWizard
- Document and View Classes
- Document Templates
- SDI and MDI Applications
- Document/View Program Structure
- Synchronizing Document and Views
- Making a Document Persistent
- Resources in Windows Programs
- Developer Studio Resource Editors
- String Tables
- Menus and Command Messages
- Update Command UI Messages
- Keyboard Accelerators
- Modal and Modeless Dialog Boxes
- Resources and Controls
- Controls as Child Windows
- CDialog Class and Programming a Modal Dialog
- Designing Dialogs with Dialog Editor
- Using ClassWizard
- Dialog Data Transfer
- Command Messages
- MFC Control Bar Classes
- Toolbars
- Idle Time Processing
- Tooltips
- Status Bars
Reviews
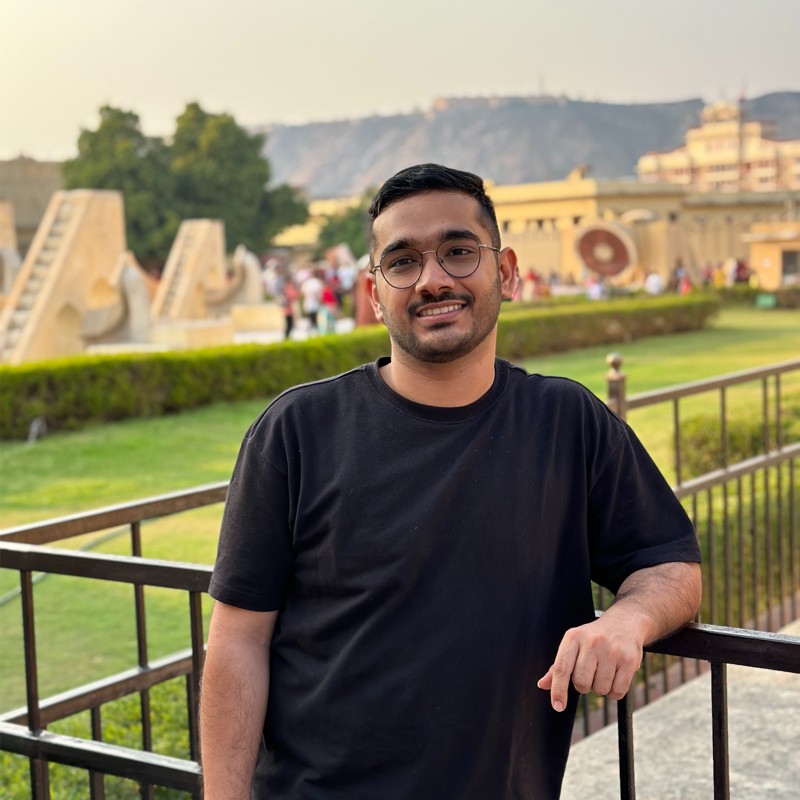
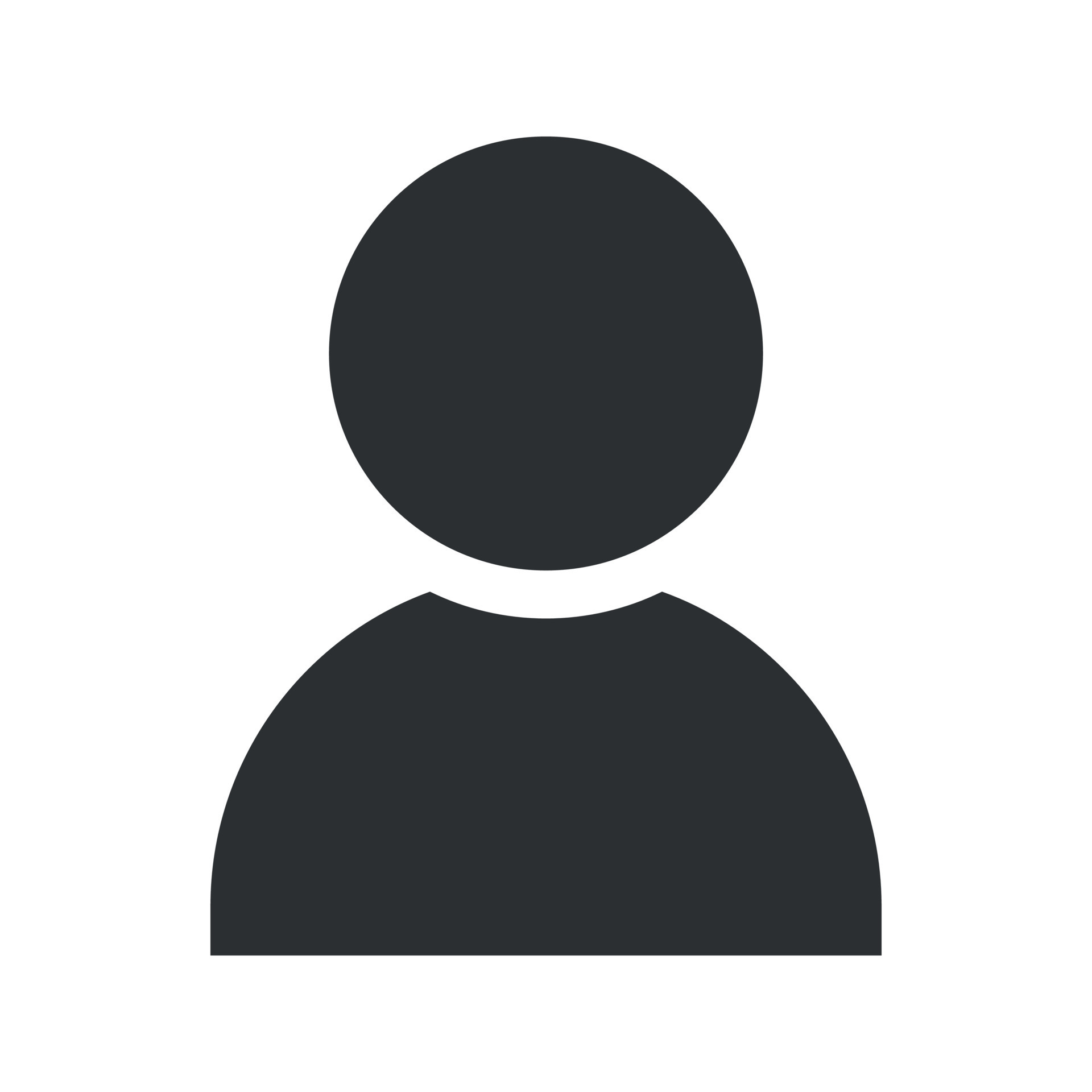
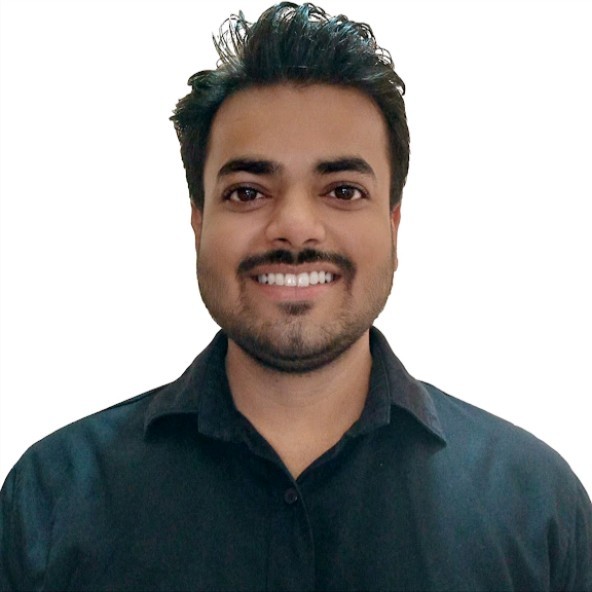
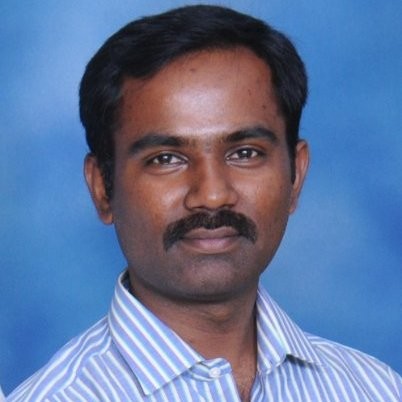
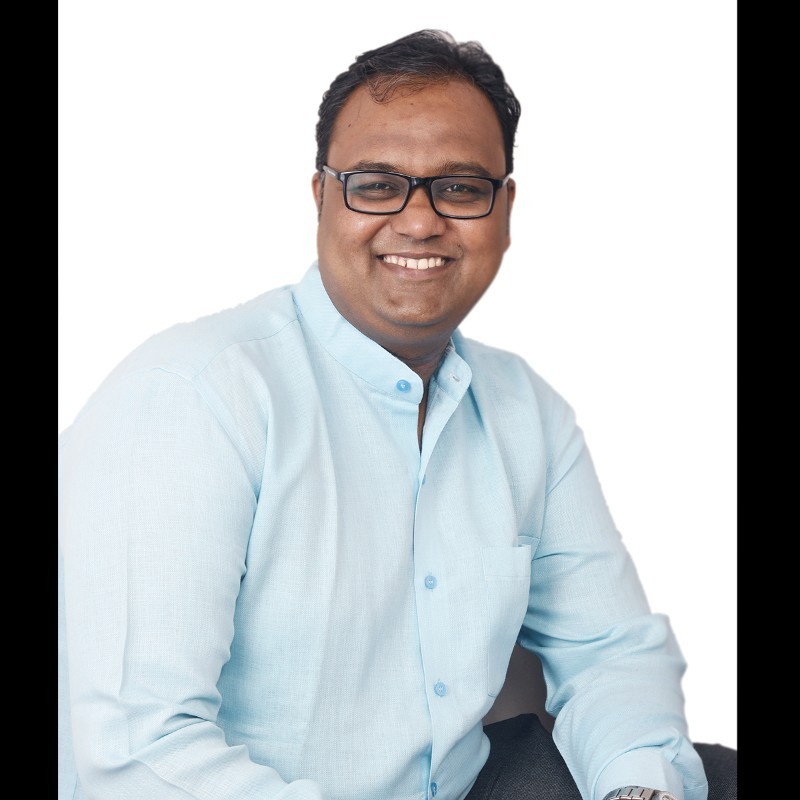
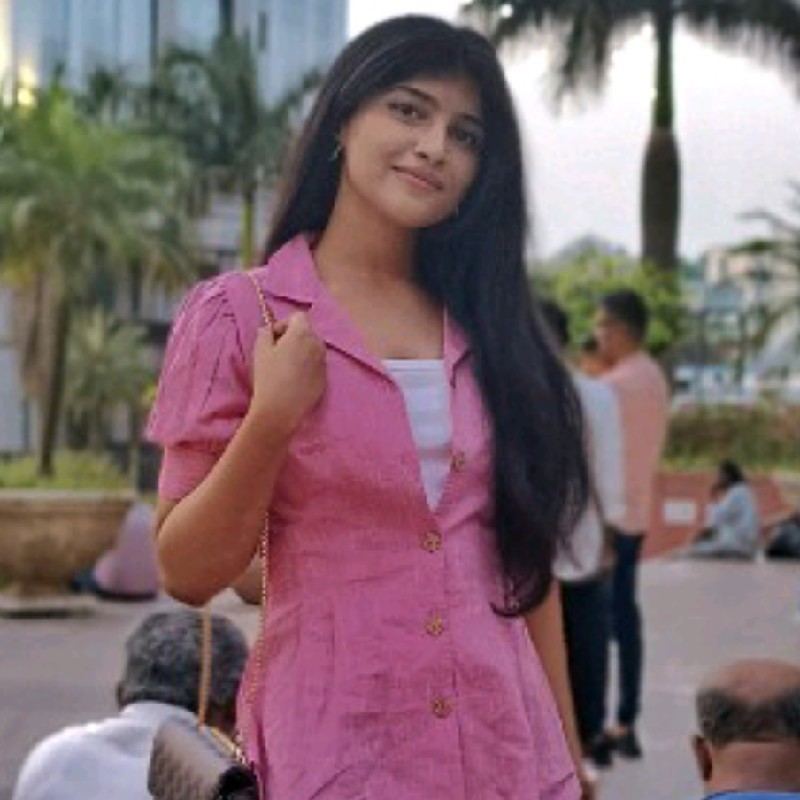
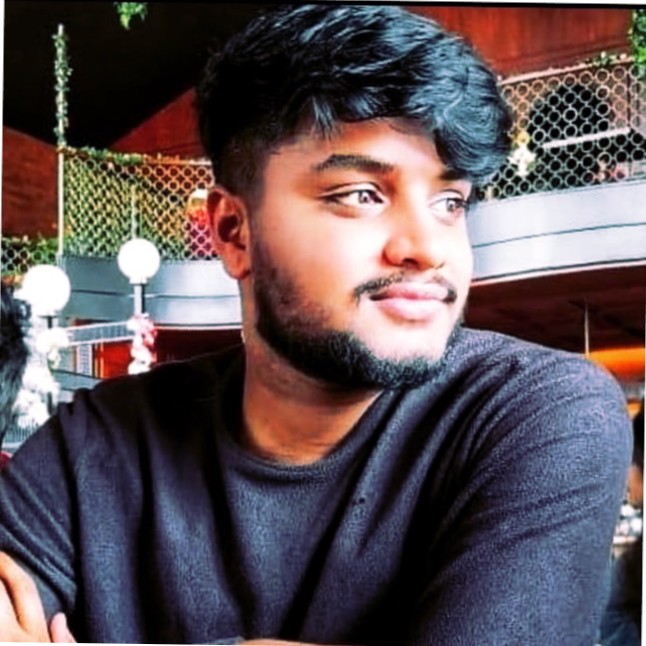
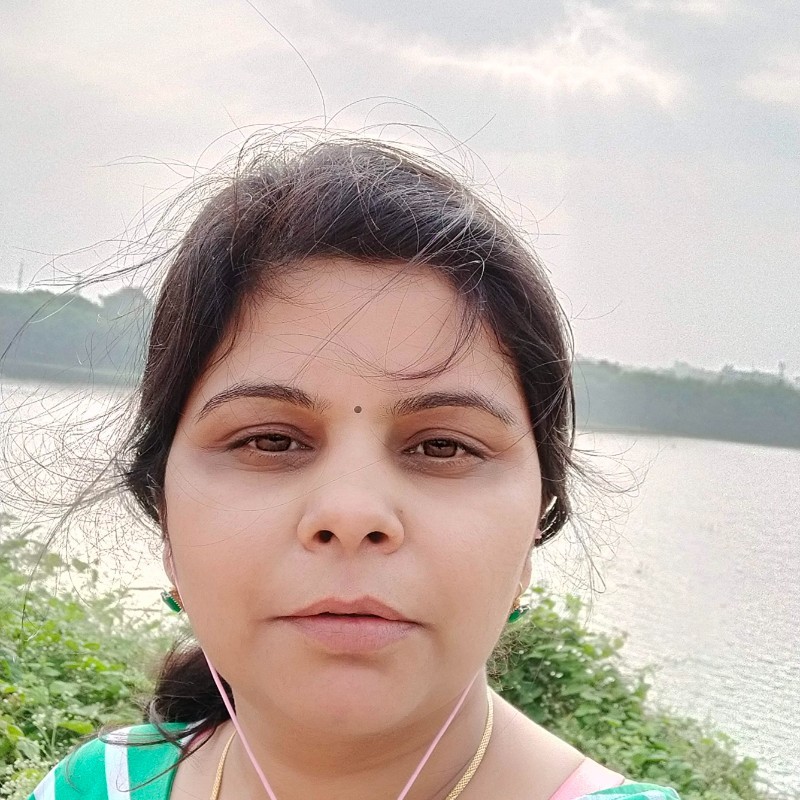
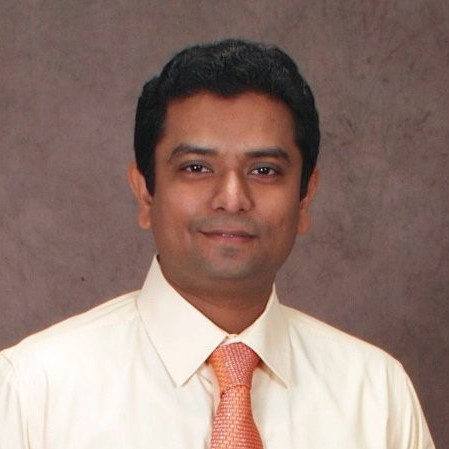
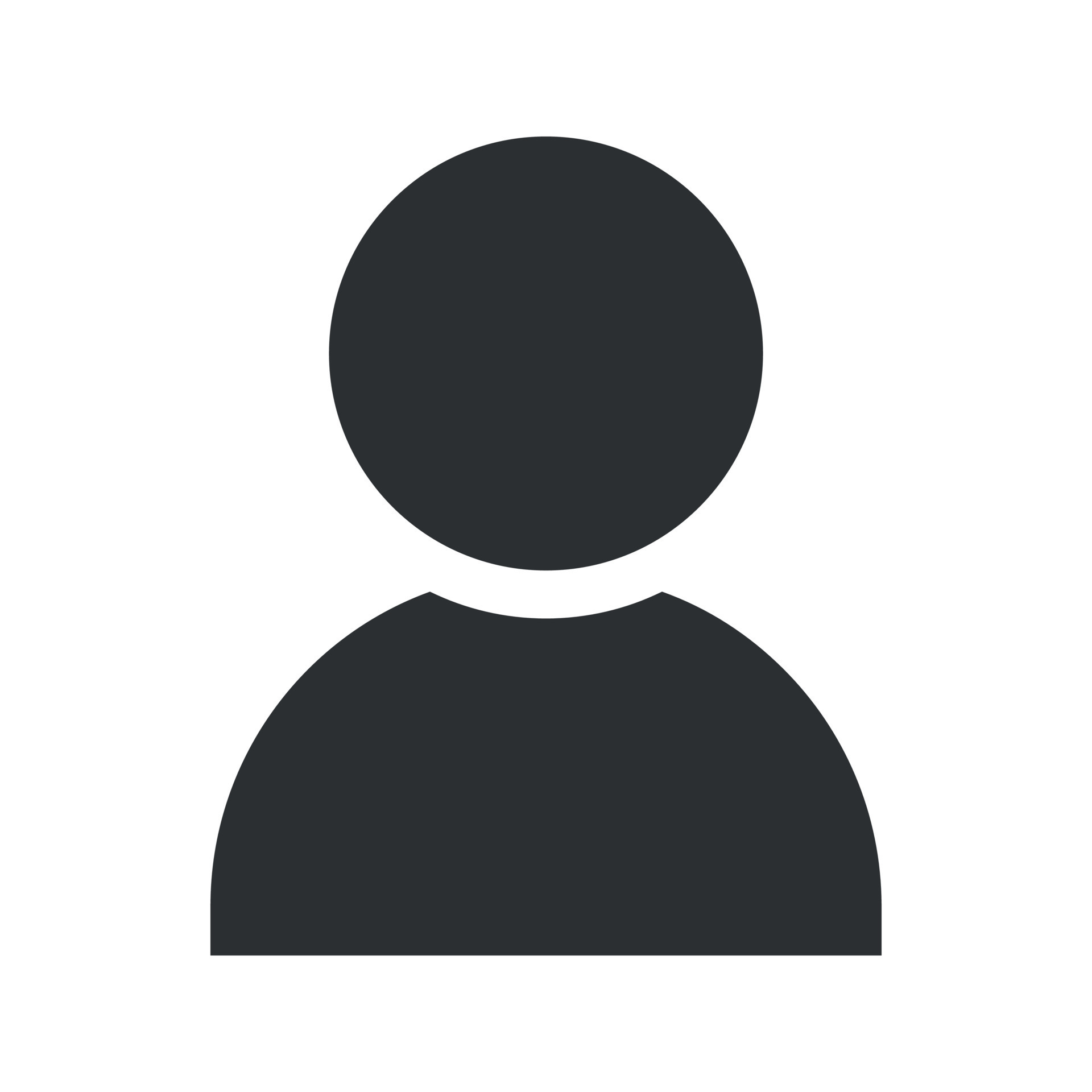
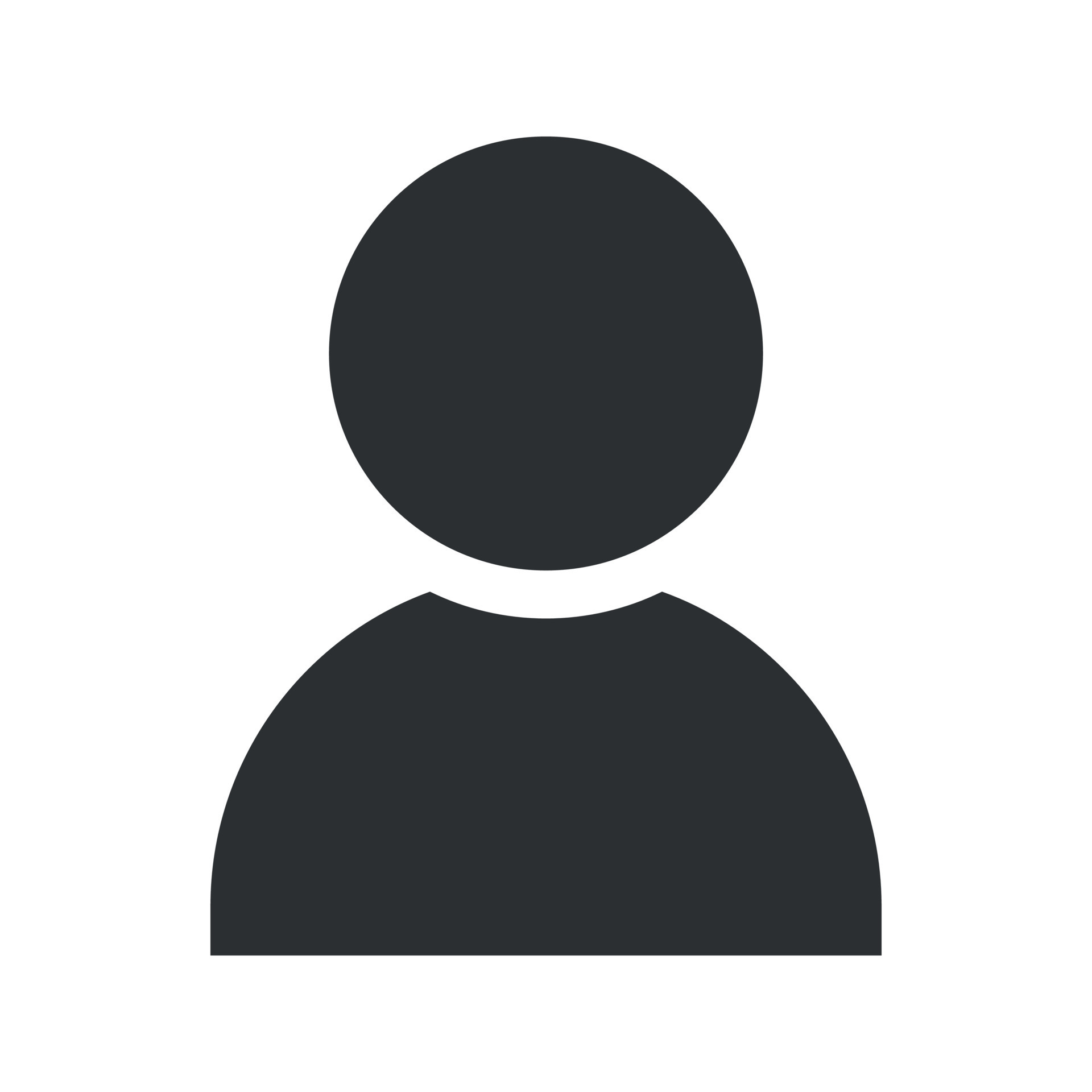
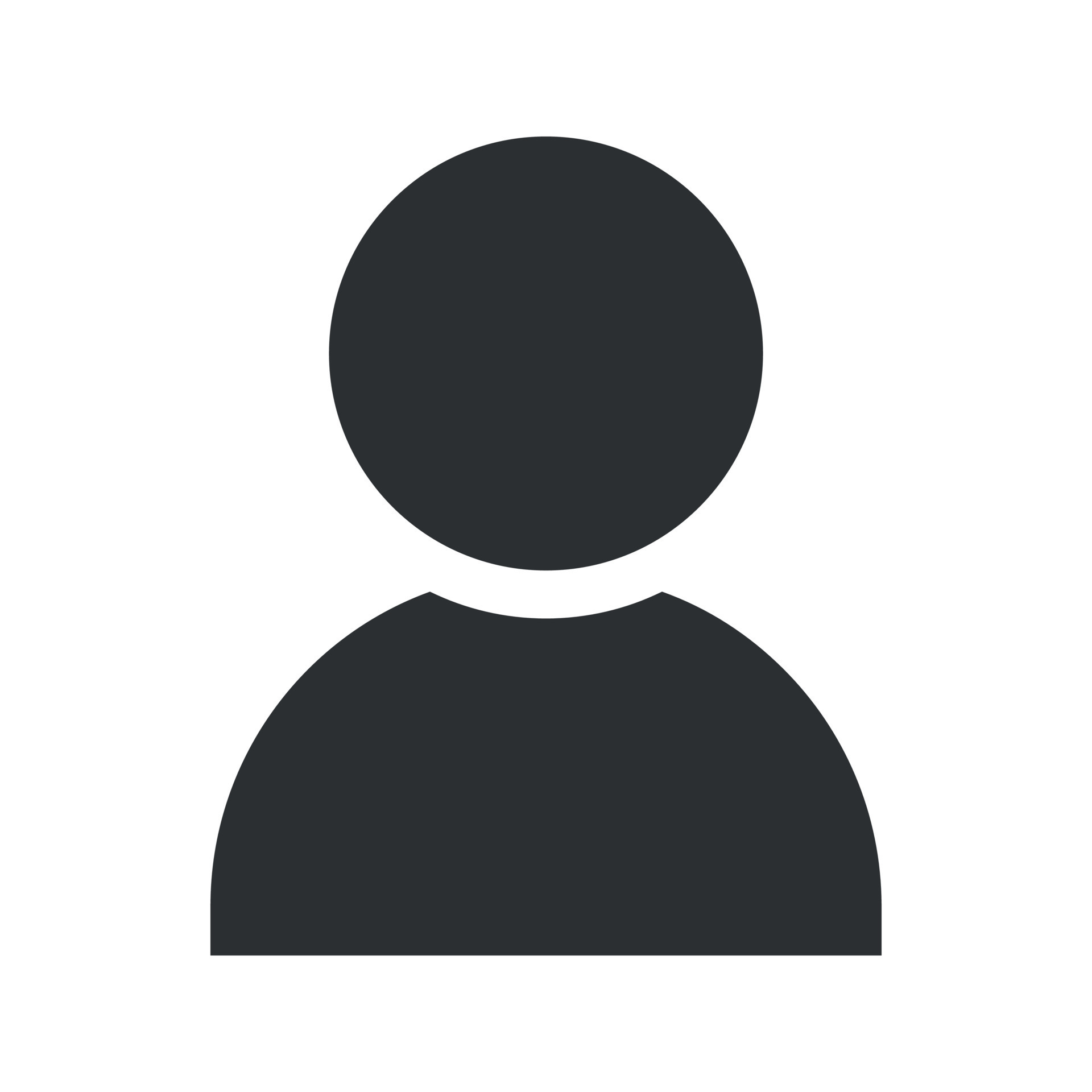
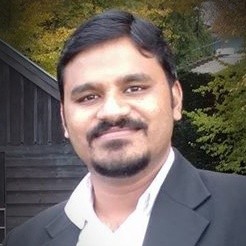
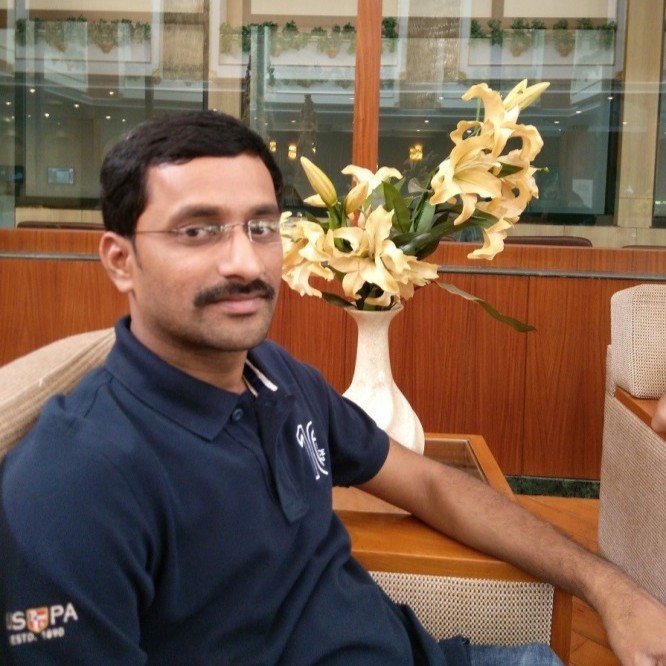
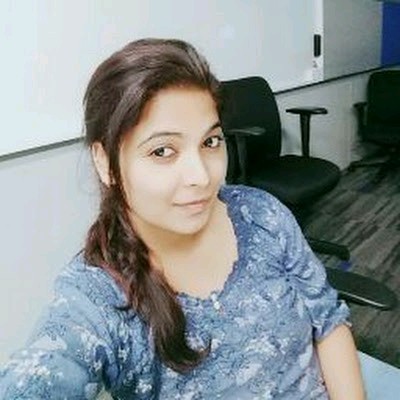