Delphi
CloudLabs
Projects
Assignment
24x7 Support
Lifetime Access
.
Course Overview
Delphi XE6 is the fastest way to develop true native applications for Windows, Mac, Android and iOS from a single codebase. Develop multi-device applications 5x to 20x faster with a proven visual development environment, component framework with source code and full access to platform APIs. Extend your existing Windows applications with mobile companion appsDelPhi is a scientific application which calculates electrostatic potentials in and around macromolecules and the corresponding electrostatic energies. It incorporates the effects of ionic strength mediated screening by evaluating the Poisson-Boltzmann equation at a finite number of points within a three-dimensional grid box.
At the end of the training, participants will be able to:
Pre-requisite
A basic knowledge of Delphi is assumed, although we do cover a primer on the language.
Duarion
5 days
Course Outline
- Introduction to Object Pascal and the Delphi programming language
- Overview of the Delphi IDE
- Delphi programming language rules
- Object Oriented System Design
- Understanding the object-oriented approach
- Programming Objectives and Application Design Steps
- Creating your first application
- Pascal identifiers, reserved words, keywords
- Understanding and working with variables
- Conditional statements and boolean evalations
- Detailed coverage of program components and generated files
- Introduction to Object Pascal Data Types
- Variables, Constants, Resource Strings
- Understanding Locality and Scope
- Object Pascal Fundamental and Generic Types
- Object Pascal Predefined Types
- Ordinal Types (Integer, Character, Boolean, Enumerated & Subrange Types)
- Integer Types examined (Byte, Cardinal, Int64, Integer, LongInt, LongWord, ShortInt, SmallInt, Word)
- Real Types examined (Comp, Currency, Double, Extended, Real, Real48, Single)
- Step-by-step application example
- Understanding the logic flow
- Analyzing the workflow and the User Interface
- Adding validations to your Delphi application
- Testing your Delphi Pascal code
- Is it bug free? How to uncover hidden problems
- Debugging your application – Stepping through your code
- Range boundaries application example
- Date and Time
- Date and Time Routines
- Calendar and Time-Tracking application example
- String Types examined (Char, AnsiChar, WideChar, ShortString, String, AnsiString, WideString)
- Working with strings
- UsingStrings application example
- Structured Types
- Working with Arrays in Delphi: Simple Arrays, Dynamic Arrays, Multi-Dimensional Arrays
- Delphi Arrays application example
- Object Pascal Record Types
- Records application example
- Object Pascal Set Types
- Sets application example
- Object Pascal File Types
- Object Pascal Pointers
- Pointers application example
- Object Pascal Variants
- Variants application example
- User-Defined Types
- Type compatibility and Typecasting
- With Statements
- Conditional Statements (If…then…else, Case)
- Repetitive Statements – Loops (For..to, For..In, While, Repeat)
- Nested Loops, Backward Loops, Multi-Path Loops
- Procedures
- Functions
- Parameters
- Passing parameters to your methods
- Step-by-step application example: Document Indexer Application – It reads a text document, parses the strings, and generates an index
- Value parameters
- Variable parameters
- Constant parameters
- Reference parameters
- Open-array parameters
- Multiple parameters
- Function Overloading
- Recursion
- Step-by-step application example: File Searching Application
- Owner vs. Parent
- Self in Delphi
- Object Pascal Classes
- Object Pascal Objects
- Dynamic Component Creation
- Inheritance
- Encapsulation
- Properties
- Scope and Visibility
- DelphiTraining Class – Using inheritance and encapsulation
- Polymorphism
- PolyHuman Class – Polymorphism in action
- Constructors
- Destructors
- Constructors and Destructors in action
- Class References
- Interfaces
- Interfaces application example
- Object Reference Model
- Overview of the Run-Time Library
- Multiple examples to demonstrate concepts and techniques
- Compile-Time Bugs
- Run-Time Bugs
- Semantic Errors
- Design Errors
- Uninitialized Variables and Objects
- Attempting to access Freed Variables and Objects
- Loops that don’t start
- Loops that don’t end (infinite loops)
- Loops that terminate before or after they should (off-by-one errors)
- Faulty Conditionals and nested IF statements
- Mathematical errors
- Range errors
- Stack overflow errors
- Understanding the Stack Memory and the Heap Memory
- Memory Leaks – What are they and how do you avoid them?
- Tools to help you debug your application
- Trapping exceptions and handling them in your application
- Creating custom exceptions
- Creating an error log
- Strategic Debugging – Learn to debug step-by-step
- Tips, tricks and techniques to use in your debugging
- Steps to make your program run well
- Multiple examples will be used to demonstrate the various concepts and techniques
Reviews
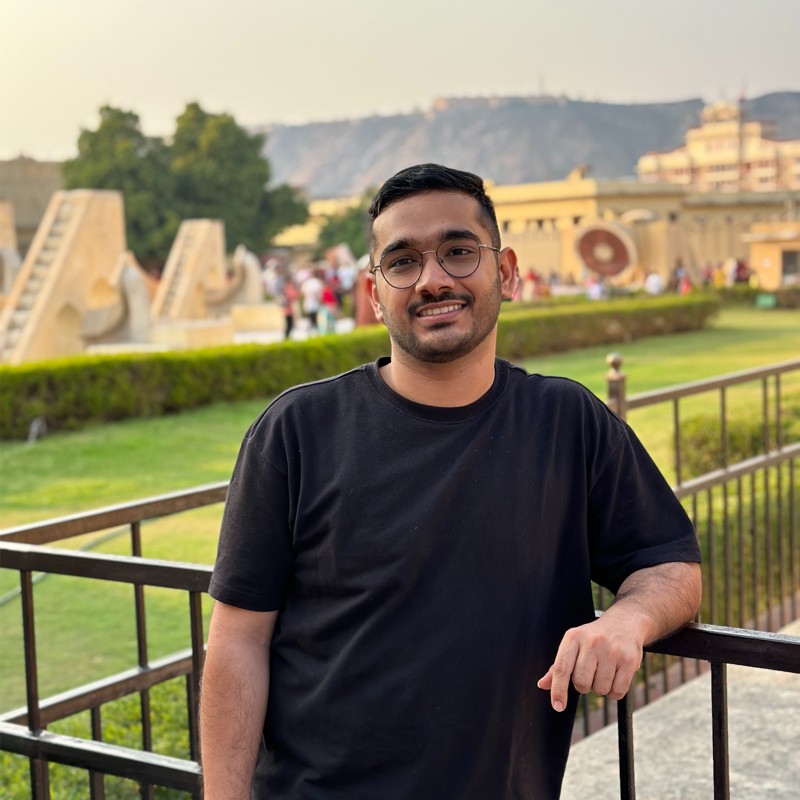
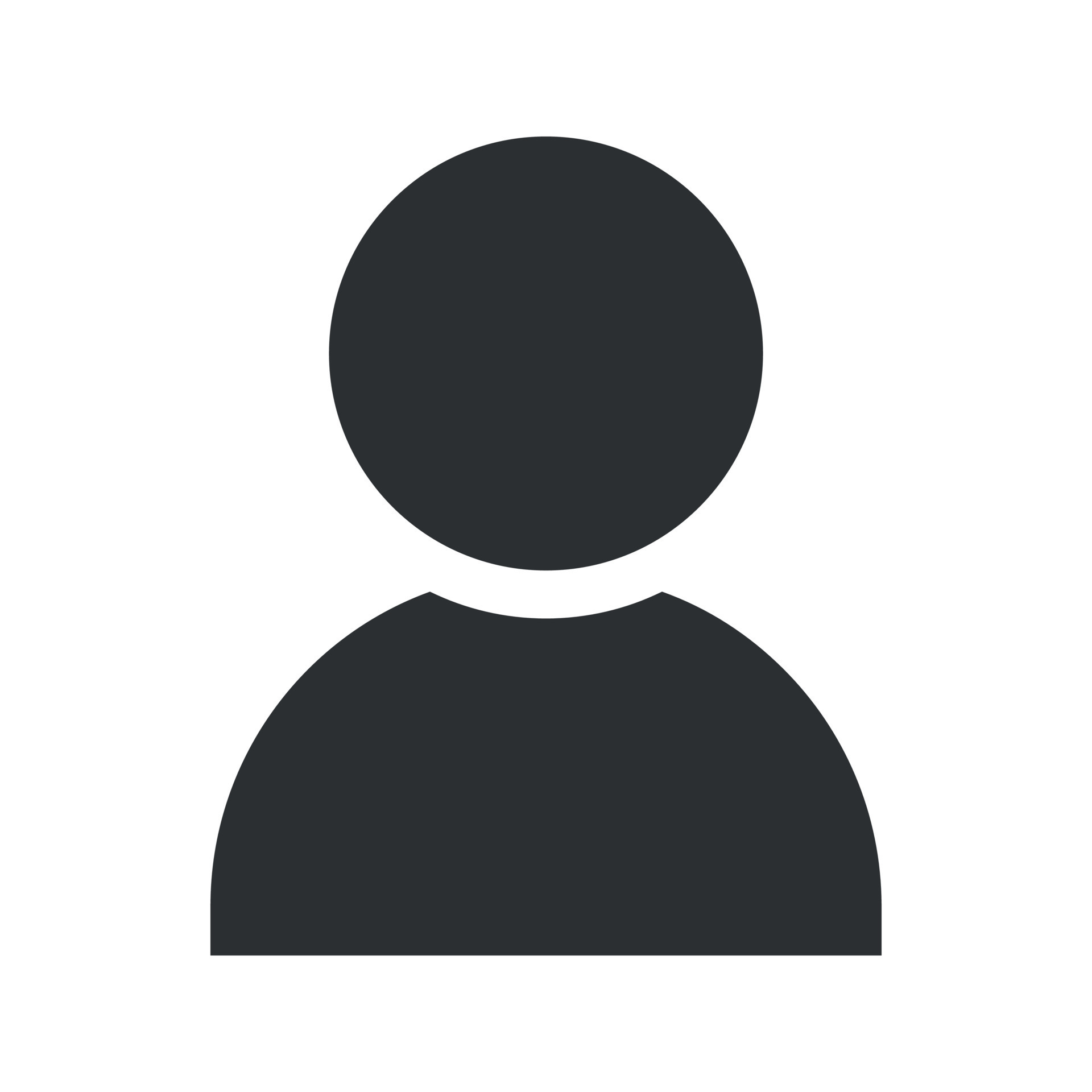
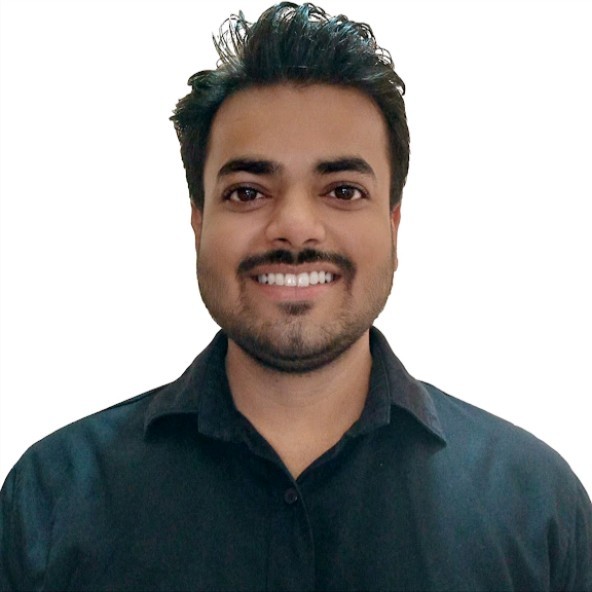
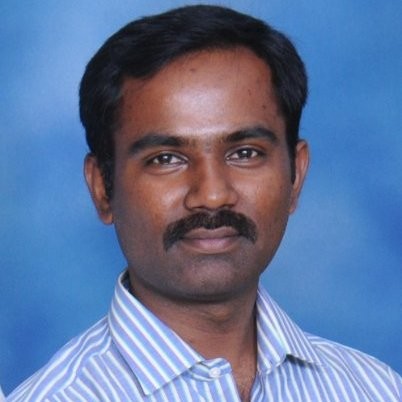
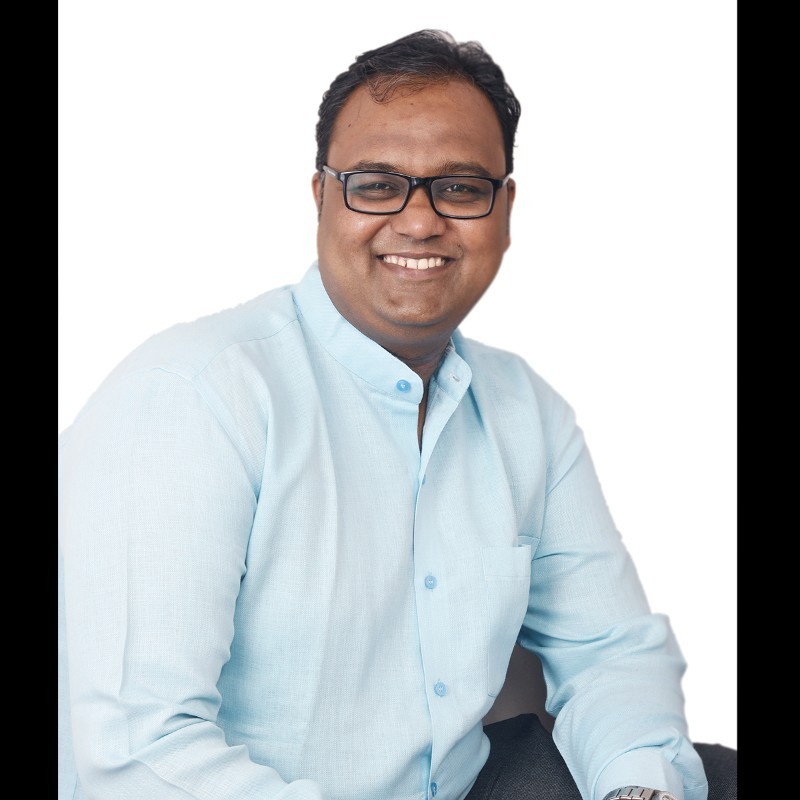
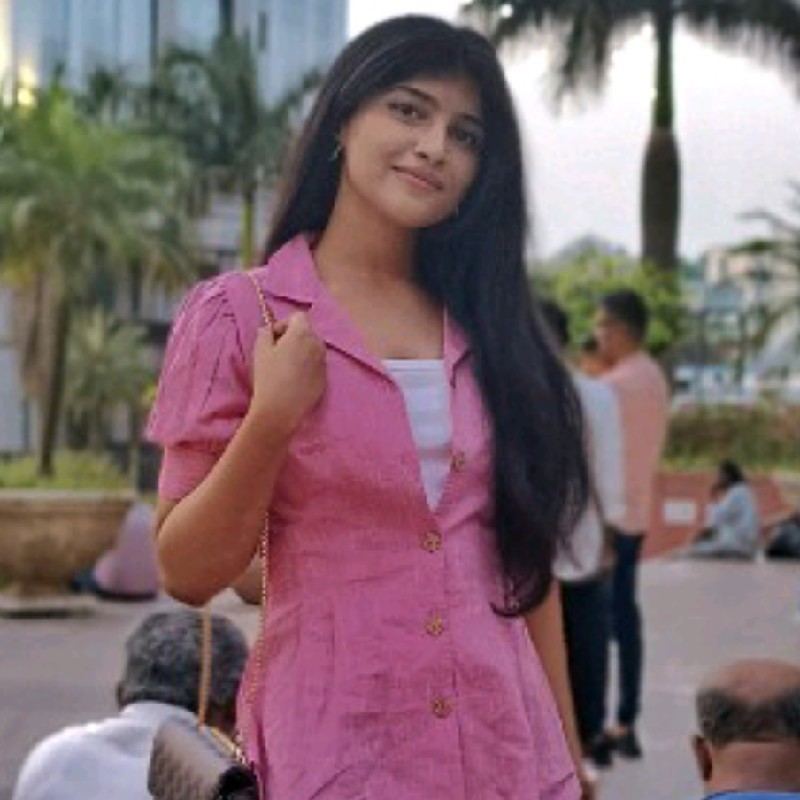
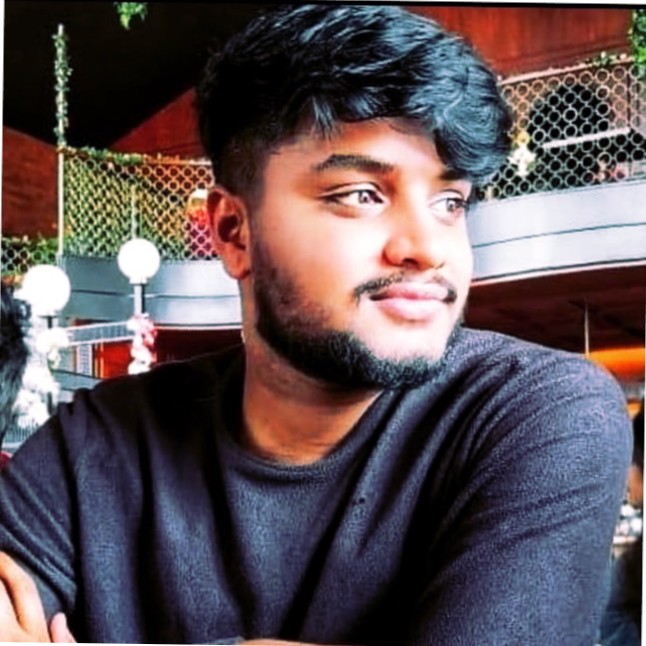
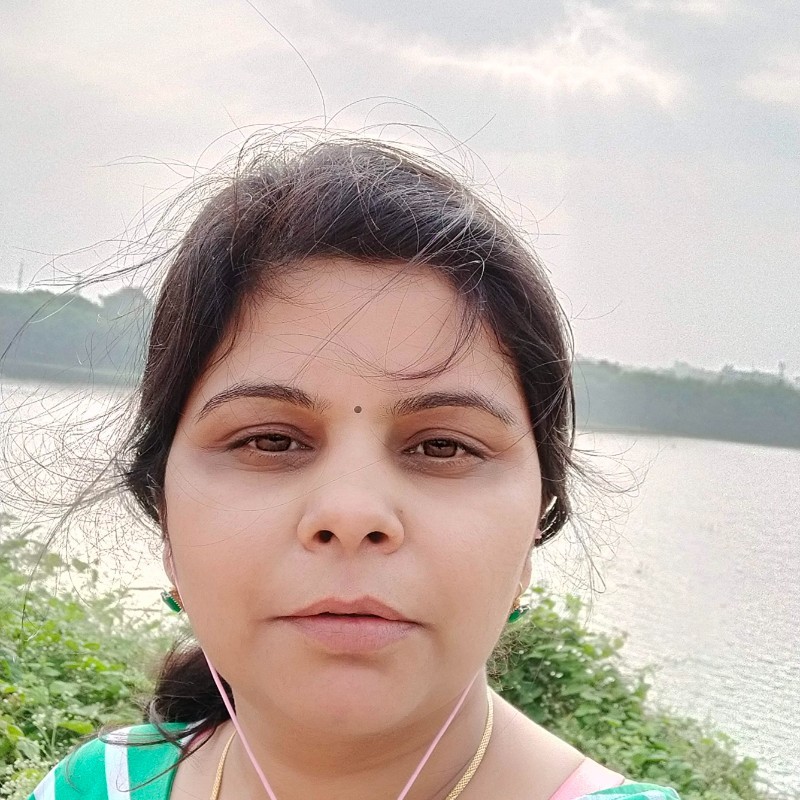
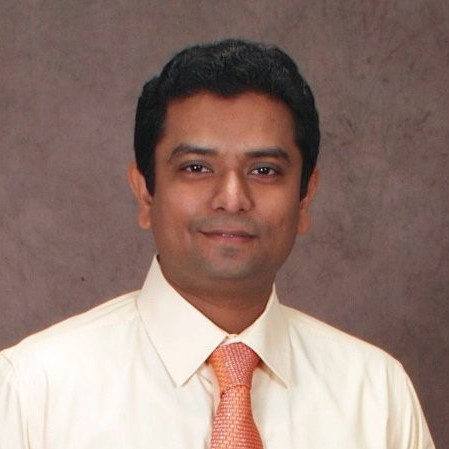
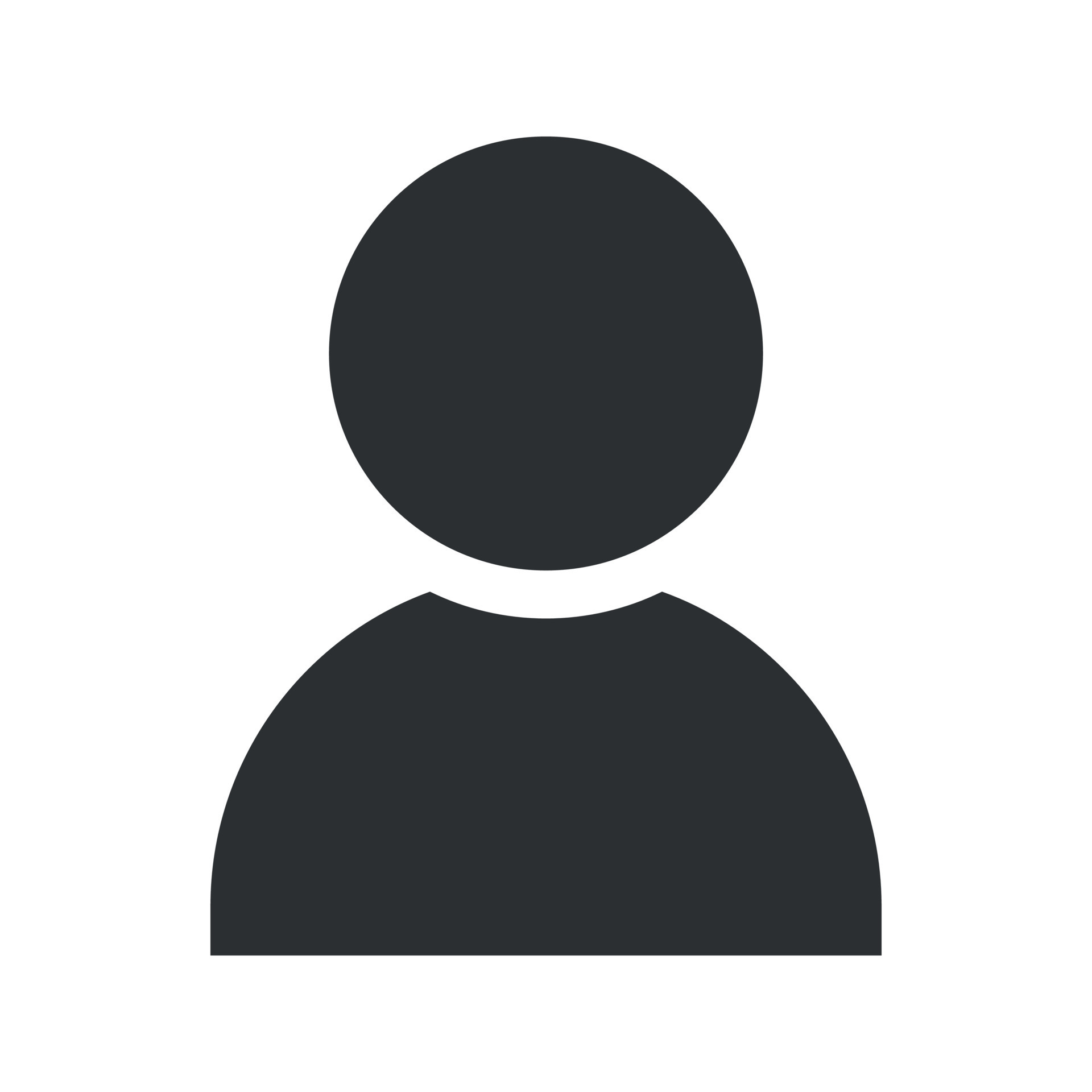
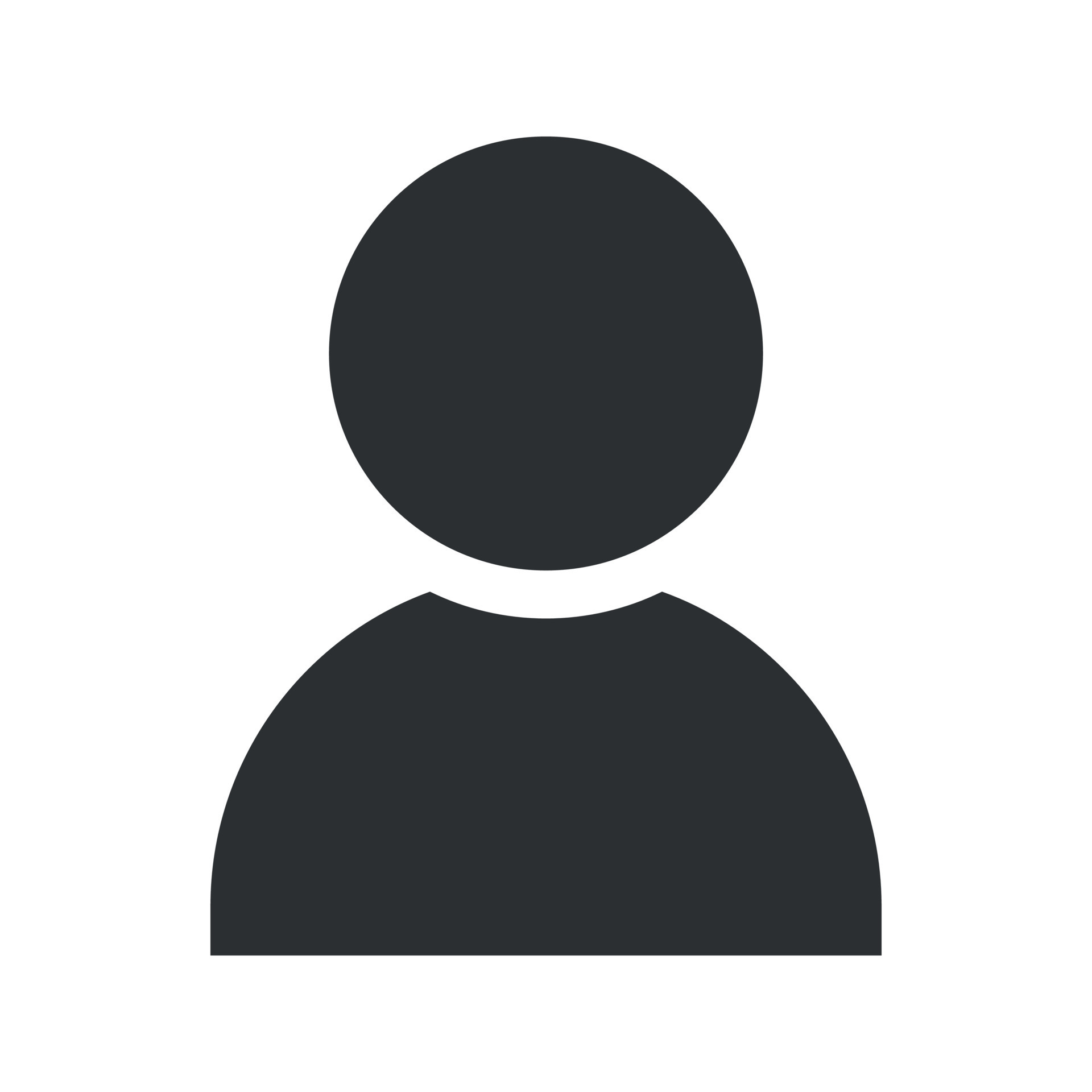
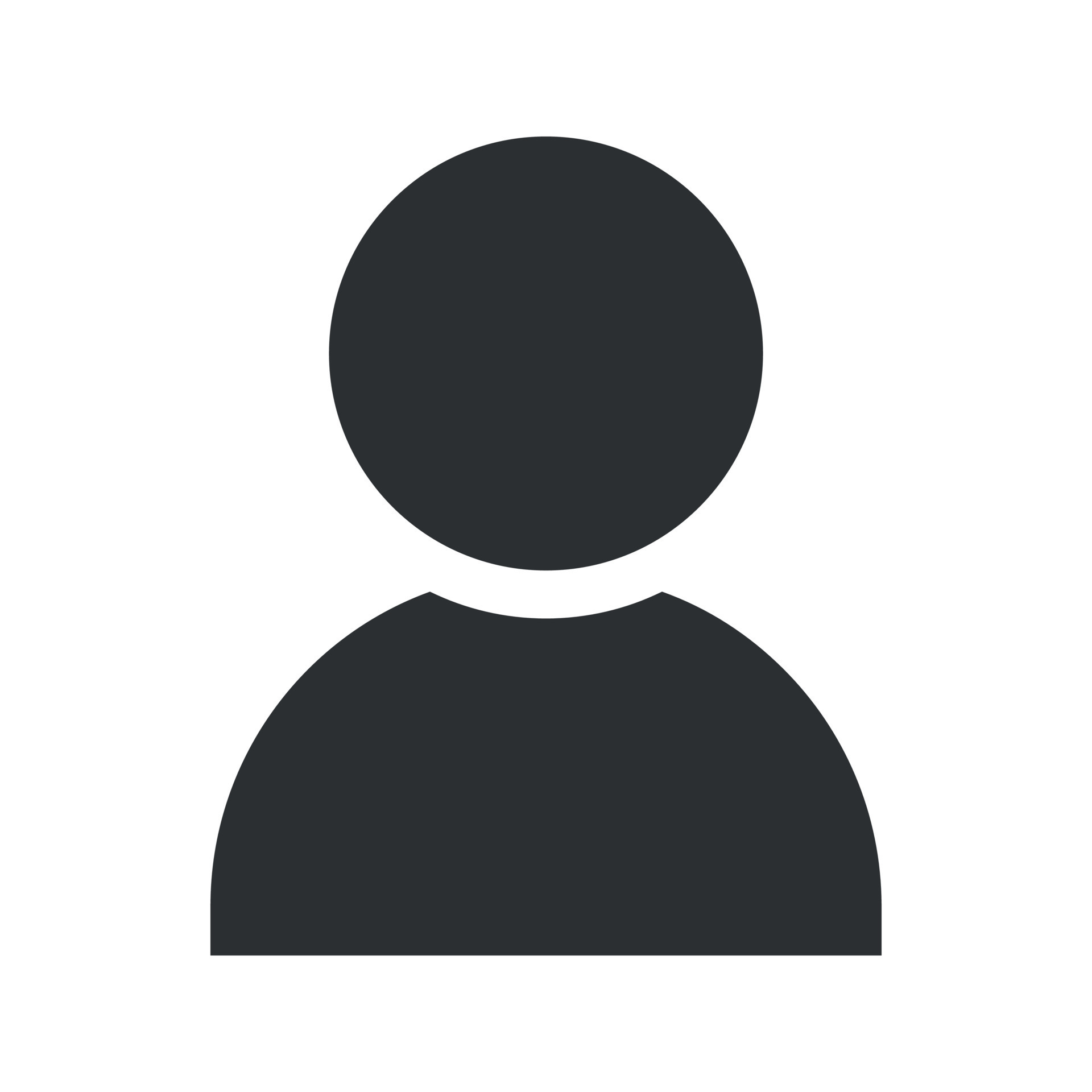
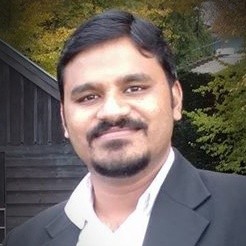
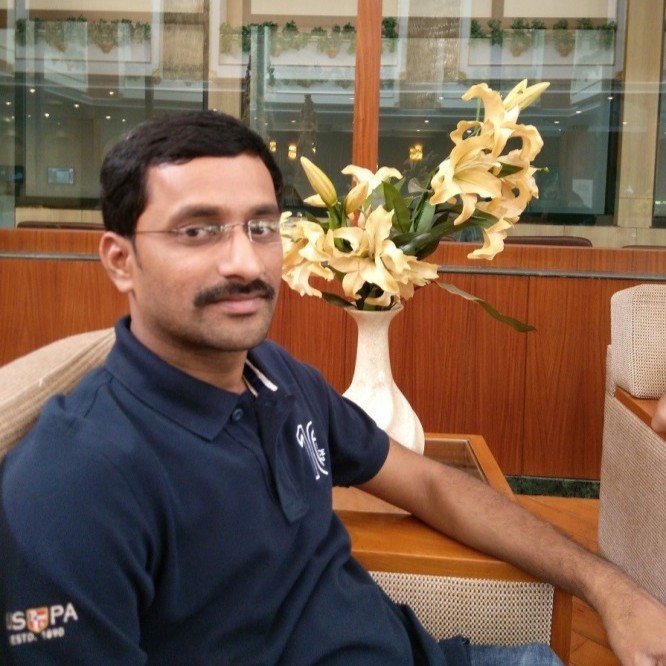
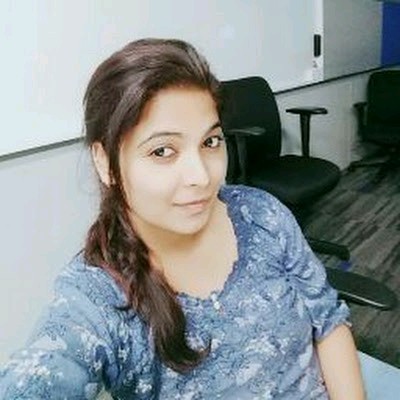