C/C++
CloudLabs
Projects
Assignment
24x7 Support
Lifetime Access
.
Course Overview
C/C++ are the general-purpose computer programming languages. Although they were designed for implementing system software, they are also widely used for developing portable application software.These includes concepts of program specification and design, algorithm development, and coding and testing using a modern software development environment.Students learn how to write programs in an object-oriented high level programming anguage.Topics covered include fundamentals of algorithms, flowcharts, problem solving, programming concepts, classes and methods, control structures, arrays, and strings.
At the end of the training, participants will be able to:
Pre-requisite
Attendees should have prior programming experience, though not necessarily in C or C++. Some prior knowledge of basic C syntax is helpful but not required
Duarion
5 days
Course Outline
- What is C?
- Background
- Sample Program
- Components of a C Program
- Examples
- Data Types
- Variables
- Naming Conventions for C Variables
- Printing and Initializing Variables
- Array Examples
- Compiling and Executing a C Program
- Examples of C Functions
- Functions
- sum Invoked from main
- Invoking Functions
- Elementary Operators
- The operator= Operators
- Operators
- The Conditional Operator
- Increment and Decrement Examples
- Increment and Decrement Operators
- Examples of Expressions
- if
- if else
- while
- for
- Endless Loops
- do while
- break and continue
- switch
- else if
- #define
- Macros
- #include
- Conditional Compilation
- #ifdef
- #ifndef
- Character I/O
- End of File
- Simple I/O Examples
- Simple I/O Redirection
- I/O with Character Arrays
- General
- Function Declarations
- Returning a Value or Not
- Function Prototypes
- Arguments and Parameters
- Organization of C Source Files
- Extended Example
- The getline Function
- The strcmp Function
- The check Function
- The atoi Function
- The average Function
- Defining the Problem Space
- A Programming Example
- Bit Wise Operators
- Bit Manipulation Functions
- Circular Shifts
- Chapter 8: Strings
- Fundamental Concepts
- Aggregate Operations
- String Functions
- Array Dimensions
- An Array as an Argument to a Function
- String Arrays
- Chapter 10: Separate Compilation
- Compiling Over Several Files
- Function Scope
- File Scope
- Program Scope
- Local static
- register and extern
- Object Files
- Libraries
- The C Loader
- Header Files
- Fundamental Concepts
- Pointer Operators and Operations
- Changing an Argument with a Function Call
- Pointer Arithmetic
- Array Traversal
- String Functions with Pointers
- Pointer Difference
- Prototypes for String Parameters
- Relationship Between an Array and a Pointer
- The Pointer Notation *p++
- Dynamic Storage Allocation – malloc
- Functions Returning a Pointer
- Initialization of Pointers
- gets – a Function Returning a Pointer
- An Array of Character Pointers
- Two Dimensional Arrays vs. Array of Pointers
- Command Line Arguments
- Pointers to Pointers
- Practice with Pointers
- Function Pointers
- Fundamental Concepts
- Describing a Structure
- Creating Structures
- Operations on Structures
- Functions Returning Structures
- Passing Structures to Functions
- Pointers to Structures
- Array of Structures
- Functions Returning a Pointer to a Structure
- typedef – New Name for an Existing Type
- Bit Fields
- unions
- Non-Homogeneous Arrays
- Enumerations
- System Calls vs. Library Calls
- Opening Disk Files
- fopen
- I/O Library Functions
- Copying a File
- Character Input vs. Line Input
- scanf
- printf
- fclose
- Servicing Errors – errno.h
- Feof
- The stat Function
- File Existence
- Telling Time – time and ctime
- Telling Time localtime
- A Database Application
- The menu Function
- The fwrite Function
- The create_db Function
- The fread Function
- The print_db Function
- fseek
- The retrieve_db Function
- fflush and ftell
- strstr
- strchr, strrchr
- system
- strtok
- strspn, strcspn
- Math Functions
- Character Testing Functions
- exit and atexit
- signal
- memcpy and memset
- qsort
- Binary Search – bsearch
- Course Prerequisites
- Course Objectives
- LCourse Delivery
- Course Practicals
- Course Structure
- Review of OOP principles
- Behaviour, state, identity,inheritance, polymorphism
- History and evolution of C++
- Key features of C++
- C++ as a better and safer C
- Grouping of data and functionality
- Syntax of a class declaration
- Syntax of use
- Public and private
- Abstract Data Types
- Program structure
- Member functions
- Function overloading
- Default arguments
- Ambiguities
- Anonymous arguments
- Resolving scope conflicts
- The Scope resolution operator
- The this pointer
- Life of an object
- Constructors
- operator new
- Death of an object
- Destructors
- operator delete
- Dynamic arrays
- Enumerations
- Const declarations
- Const member functions
- Const member data
- Inline function mechanism
- Reference variables
- An opportunity for reuse
- Scoping and initialisation
- Order of construction
- Member Initialisation lists
- Use of fundamental classes
- Delegating class functionality
- Dynamic associations
- Custody and lifetime
- Constant associations
- Operator functions
- Unary operators
- Binary operators
- Global operators
- Member operators
- Subscript operators
- Input operators
- Output operators
- Guidelines
- Static data members
- Static member functions
- Nested types
- Forward declarations
- Friend classes
- Organising collections of objects
- Template classes
- vector
- list
- Iterators
- Template functions
- Algorithms
- Using the Standard Library
- The copy assignment operator
- Copy constructors
- Conversions to a class object
- Conversions from a class object
- Extension of existing classes
- Notation, syntax, terminology
- Protected members
- Scoping and initialisation
- Multiple inheritance
- Abstract base classes
- Guidelines
- Modified class behaviour
- Virtual functions
- virtual destructors
- Late binding
- Inside the virtual function mechanism
- Pure virtual functions
- Use of pointers to base type
- Guidelines
Reviews
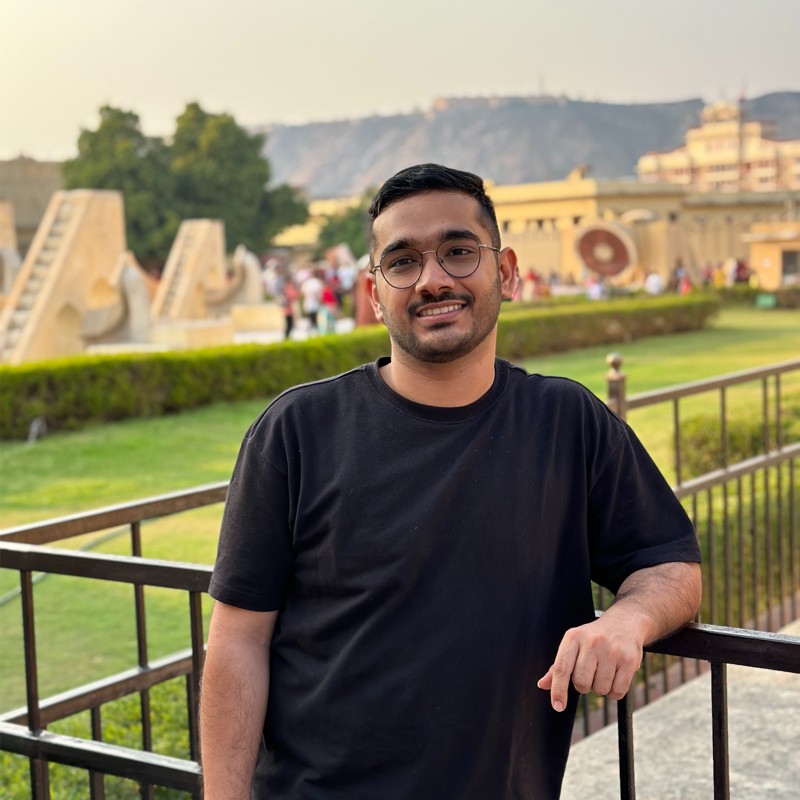
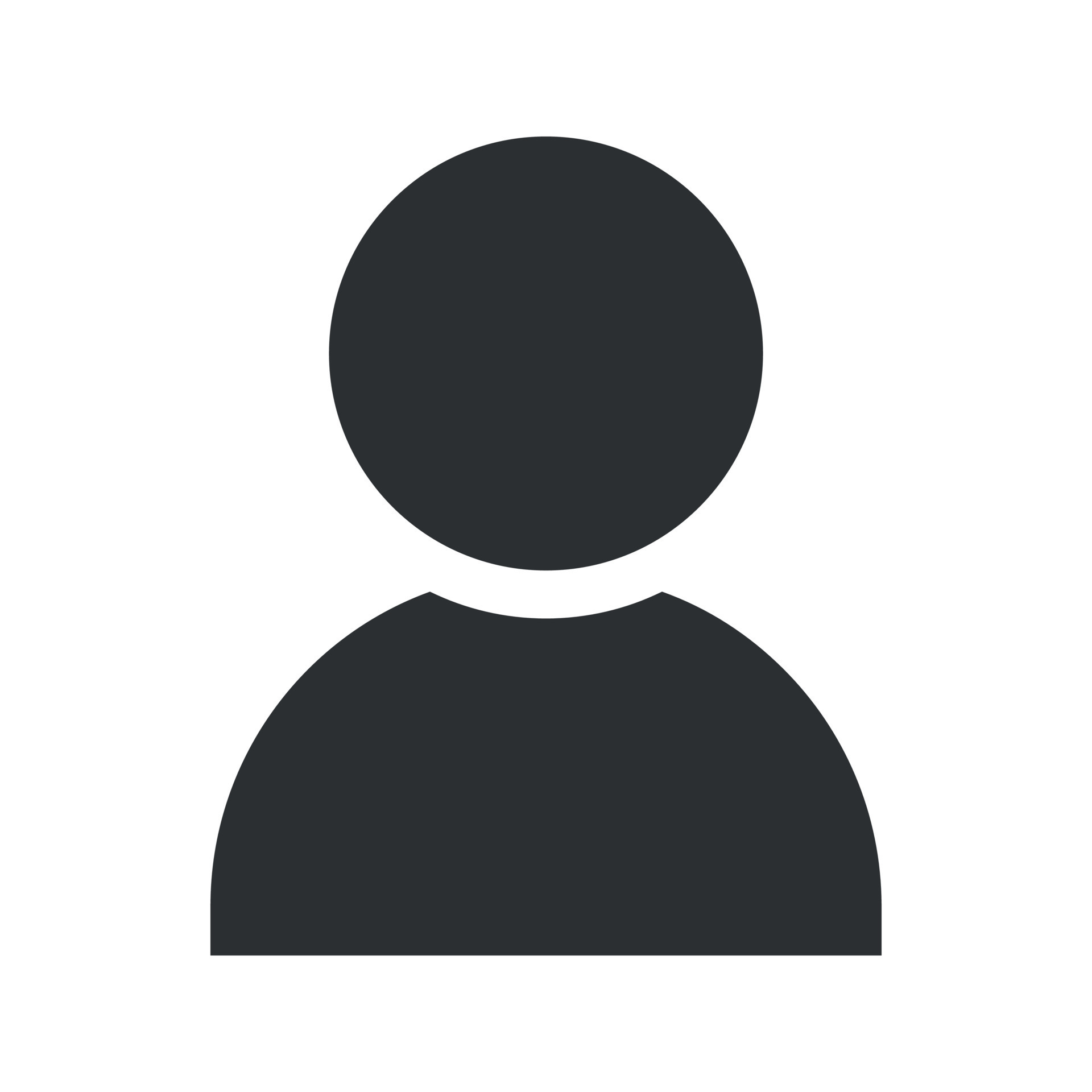
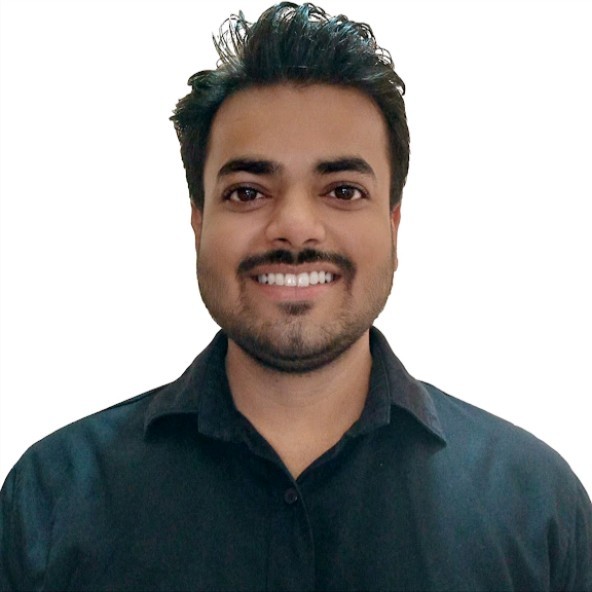
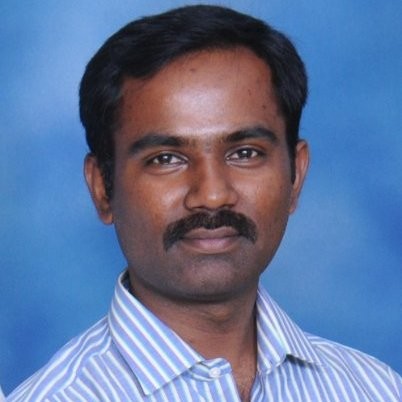
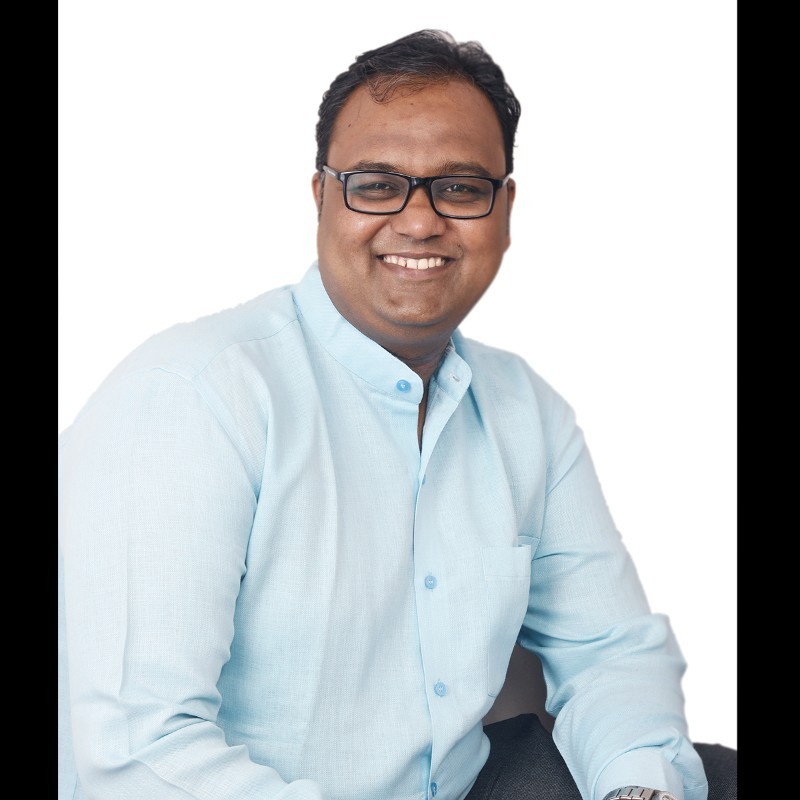
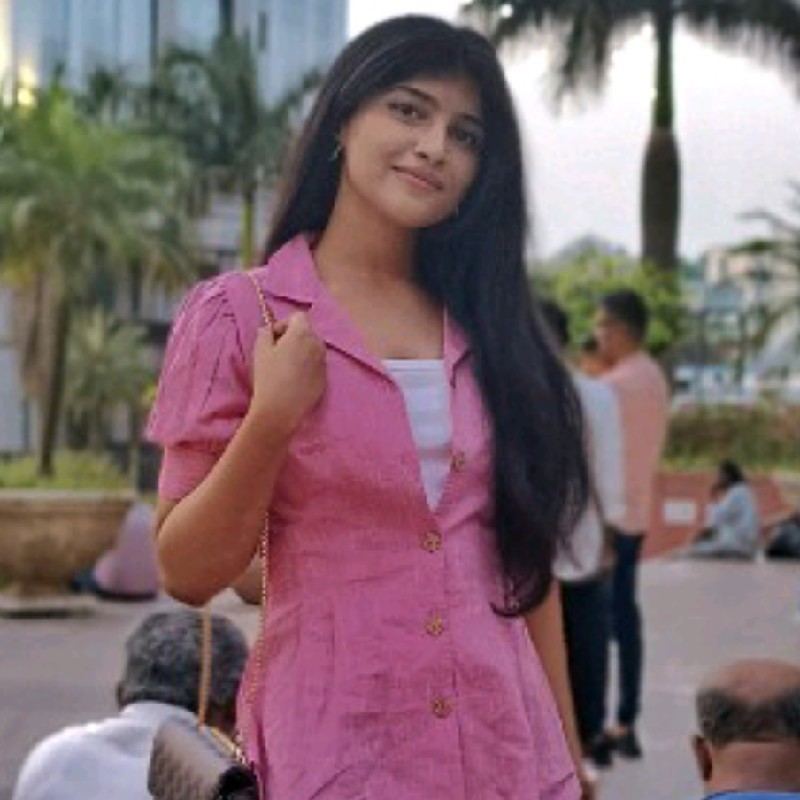
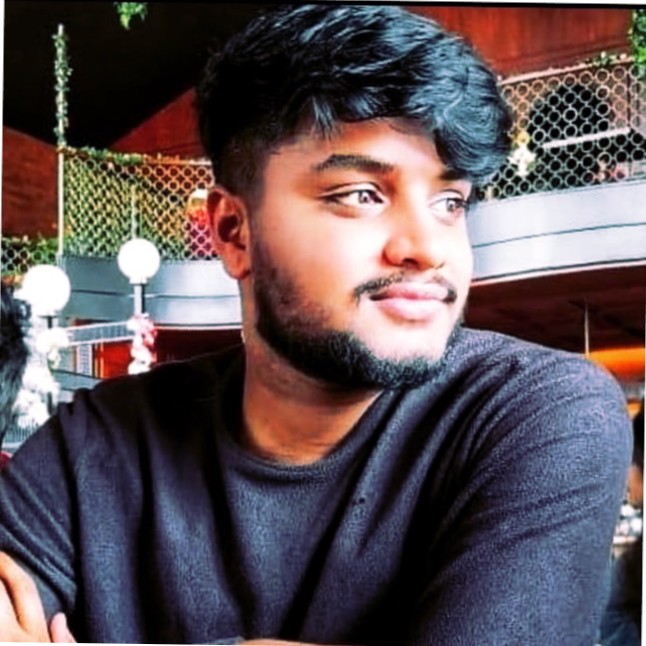
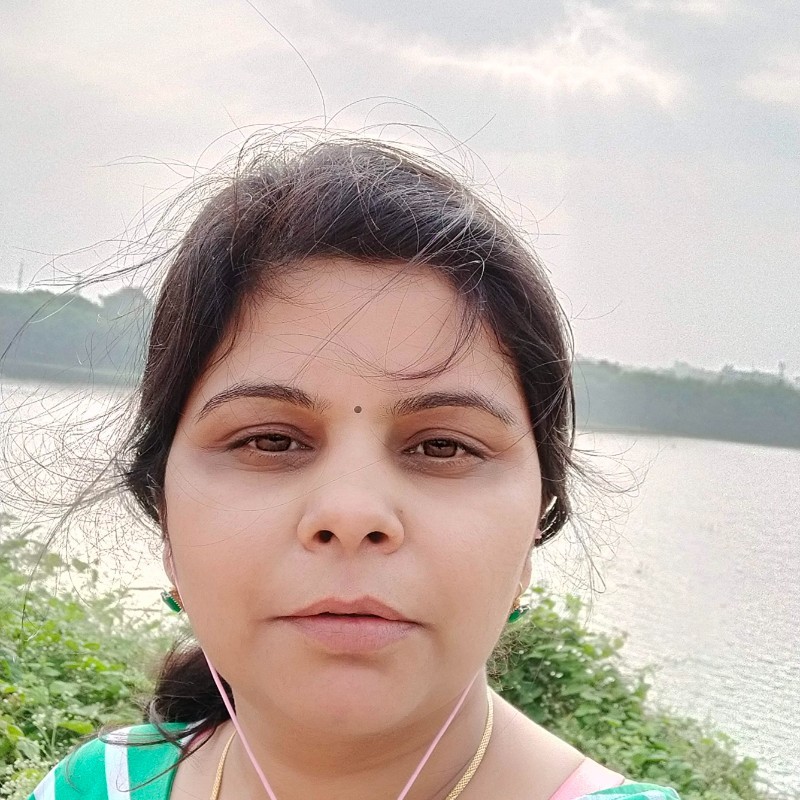
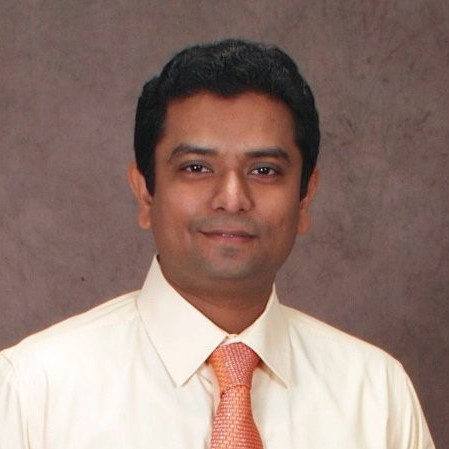
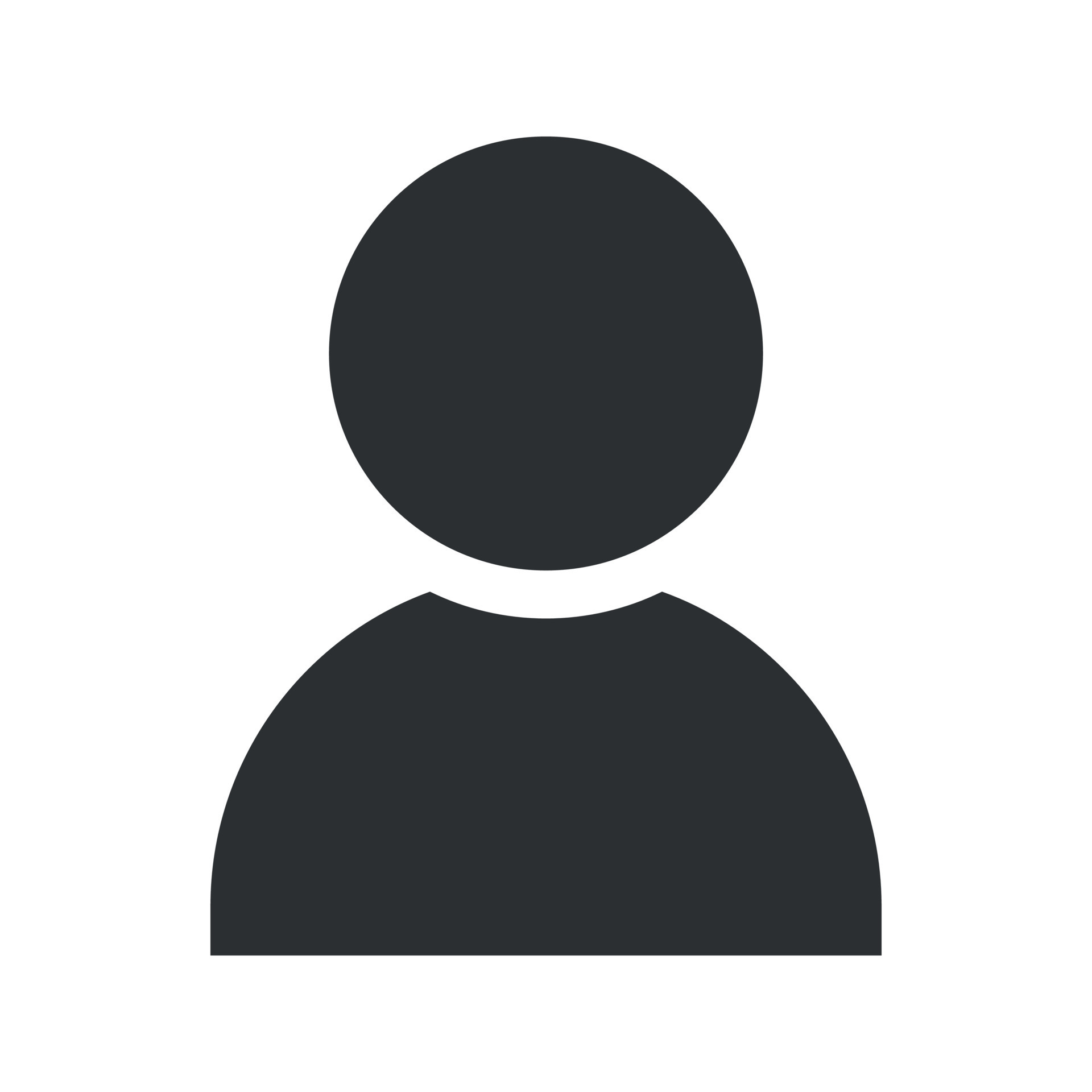
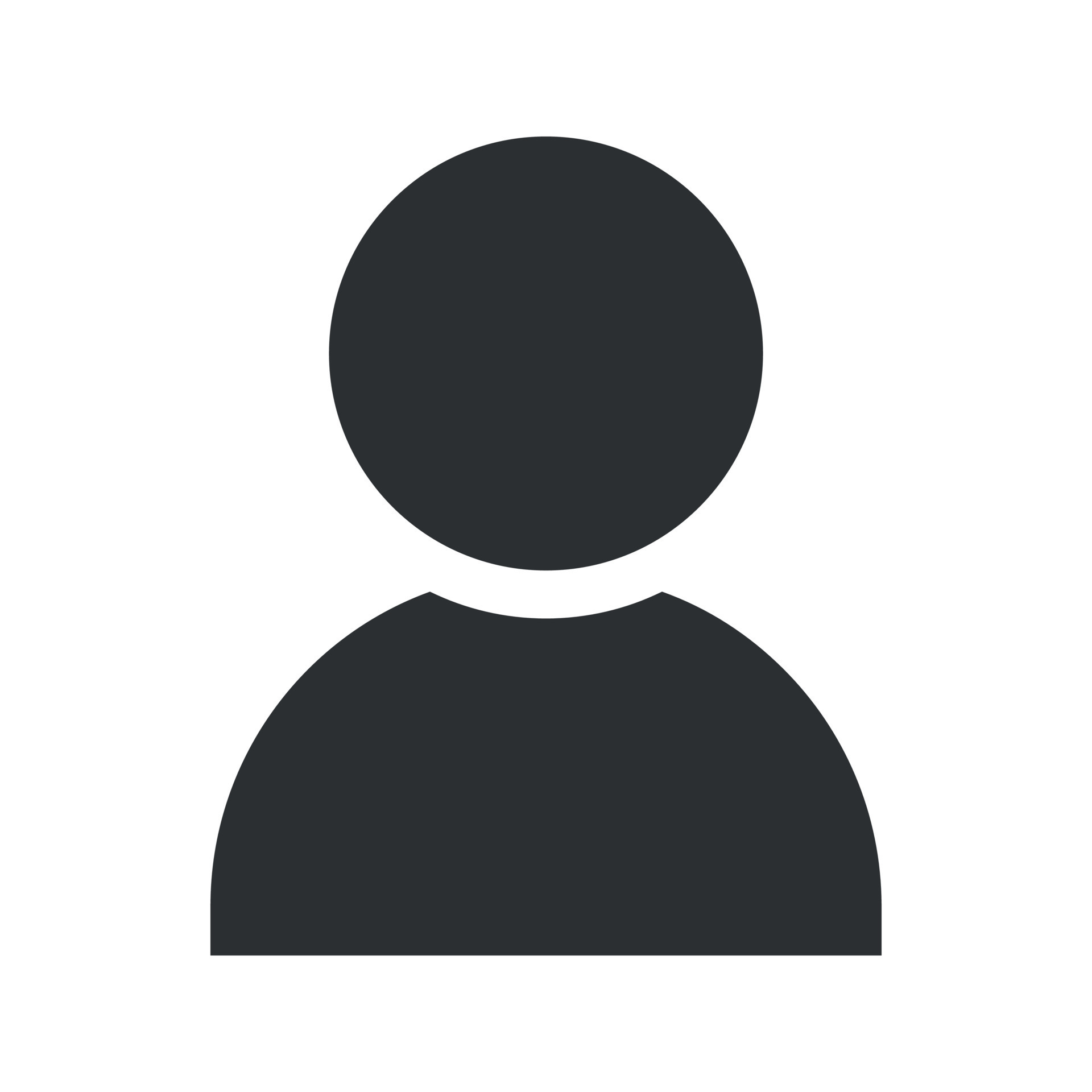
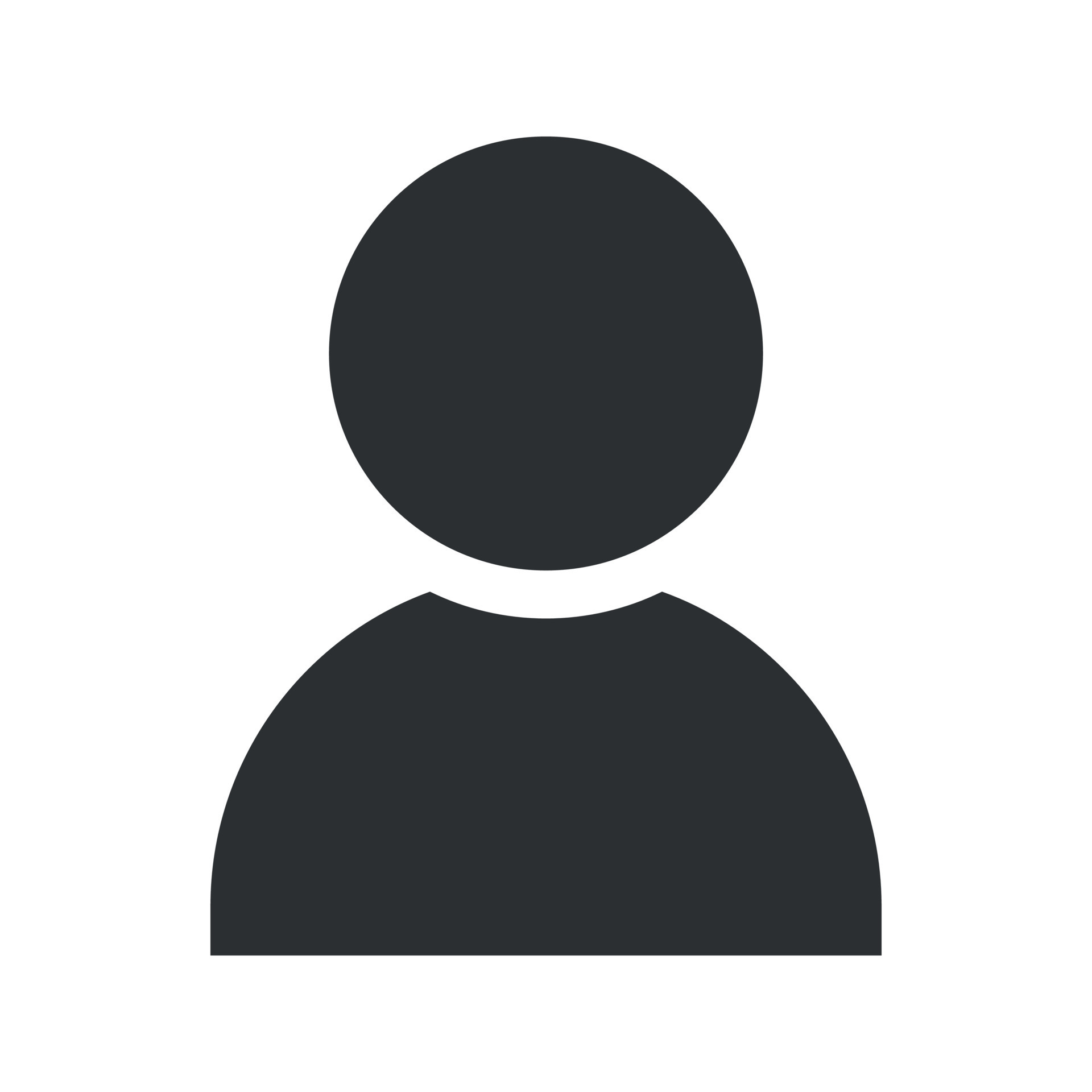
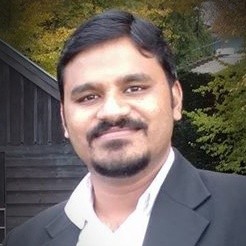
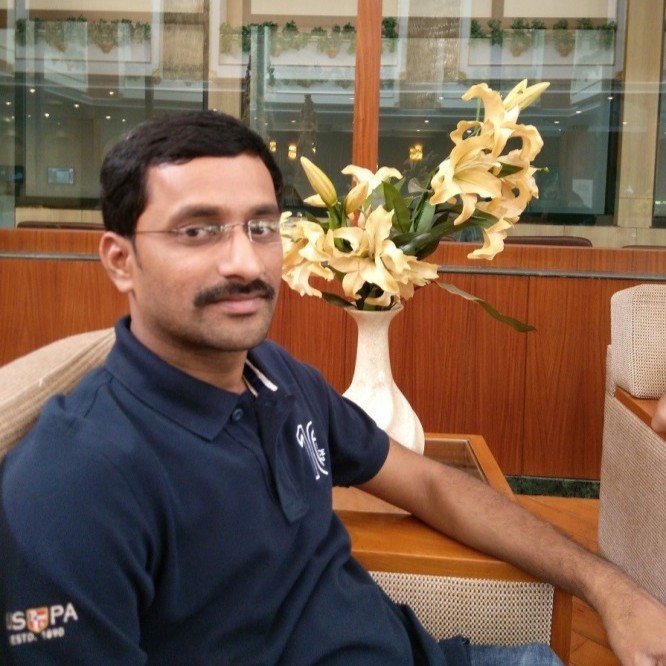
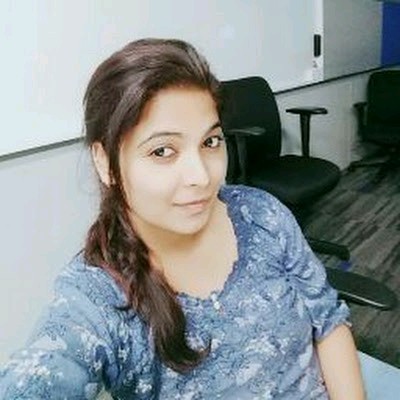