Angular 9
Course Overview
Developed and maintained by Google, Angular has evolved over the years into a comprehensive development framework that includes all of the tools and components required to build a web applications.
At the end of the training, participants will be able to:
- Introduce Angular 9
- Discuss what is Angular 9
- Discuss the various versions of Angular
- Define the features of Angular 9
- Explain about Typescript and its features
Pre-requisite
- Any developer who is building Angular applications
- Teams getting started with or working on Angular projects
Duration
5 Days
Course Outline
- Introduction
- Types & Strict Typing
- REPL
- Built-in types
- Classes
- Properties
- Methods
- Constructors
- Inheritance
- Utilities
- Annotations
- Tuples
- Fat Arrow Functions
- Template Strings
- Introduction to SPA’s & Angular
- Angular v/s v/s React v/s Vue
- Preparing the Angular-cli &Development Environment
- Creating and Preparing the Project
- Creating the Project
- Adding the Bootstrap CSS Package
- Starting the Development Tools
- Editing the HTML File
- Adding Angular Features to the Project
- Preparing the HTML File
- Creating a Data Model
- Preparing the Template
- Preparing the Component
- Understanding DI (dependency injection)
- Putting the Application Together
- Adding Features to the Example Application
- Adding the To-Do Table
- Creating a Two-Way Data Binding
- Adding To-Do Items
- Finishing touches
- Preparing the Project
- Installing the Additional NPM Packages
- Preparing the RESTful Web Service
- Preparing the HTML File
- Understanding the MVC Pattern
- Understanding RESTful Services
- Common Design Pitfalls
- Putting the Logic in the Wrong Place
- Adopting the Data Store Data Format
- Planning Architecture (SRP / Separation of concerns )
- Starting the RESTful Web Service
- Preparing the Angular Project Features
- Updating the Root Component
- Updating the Root Module
- Inspecting the Bootstrap File
- Starting the Data Model
- Creating the Model Classes
- Creating the Data Source with Observables
- Creating the Model Repository
- Creating the Feature Module
- Creating the Store Component and Template
- Creating the Store Feature Module
- Updating the Root Component and Root Module
- Adding Store Features the Product Details
- Displaying the Product Details
- Adding Category Selection
- Adding Product Pagination
- Creating a Custom Directive
- Understandind SRP & OCP etc (single responsibility principle & Open & Closed Principle)
- Creating the Cart
- Creating the Cart Model
- Creating the Cart Summary Components
- Integrating the Cart into the Store
- Adding URL Routing
- Creating the Cart Detail and Checkout Components
- Creating and Applying the Routing Configuration
- Navigating Through the Application
- Guarding the Routes
- Completing the Cart Detail Feature
- Processing Orders
- Extending the Model
- Collecting the Order Details
- Using the RESTful Web Service
- Applying the Data Source
- View Encapsulation
- Creating the Lazy Loaded Module
- Configuring the URL Routing System
- Navigating to the Administration URL (Auxiliary routes & Lazy Loading)
- Implementing Authentication
- Understanding the Authentication System
- Extending the Data Source
- Creating the Authentication Service
- Enabling Authentication
- Extending the Data Source and Repositories
- Creating the Administration Feature Structure
- Creating the Placeholder Components
- Preparing the Common Content and the Feature Module
- Implementing the Product Feature
- Rxjs Operators
- Implementing the Orders Feature
- Adding Progressive Features
- Installing the PWA Package
- Caching the Data URLs
- Responding to Connectivity Changes
- Preparing the Application for Deployment
- Creating the Data File
- Creating the Server
- Changing the Web Service URL in the Repository Class
- Building and Testing the Application
- Testing the Progressive Features
- Containerizing the SportsStore Application
- Installing Docker
- Preparing the Application
- Creating the Docker Container
- Running the Application
- Adding Form Data Validation
- Validating the Entire Form
- Using Model-Based Forms
- Enabling Model-Based Forms Feature
- Defining the Form Model Classes
- Using the Model for Validation
- Generating the Form Elements from the Model / Service
- Creating Custom Form Validators
- Applying a Custom Validator
- Creating a Simple Attribute Directive
- Applying a Custom Directive
- Accessing Application Data in a Directive
- Reading Host Element Attributes
- Creating Data-Bound Input Properties – @input
- Responding to Input Property Changes
- Creating Custom Events – @output
- Binding to a Custom Event
- Creating Host Element Bindings – @hostBinder & @hostListener
- Creating a Two-Way Binding on the Host Element
- Replacing the ngModel directive
- Exporting a Directive for Use in a Template Variable
- Change Detection Strategy
- Creating a Simple Structural Directive
- Implementing the Structural Directive Class
- Enabling the Structural Directive
- Using the Concise Structural Directive Syntax
- Creating Iterating Structural Directives
- Replacing the *ngFor directive
- Providing Additional Context Data ( Template variables like index,first,last,odd,even etc)
- Using the Concise Structure Syntax
- Dealing with Property-Level Data Changes – ngOnInit & ngOnChanges
- Dealing with Collection-Level Data Changes – ngDoCheck
- Restricting Memory leak – ngDoCheck
- Querying the Host Element Content @ViewChild
- Querying Multiple Content Children @ViewChildren
- Receiving Query Change Notifications
- Understanding the Performance issue with ngDoCheck
- Solving the Problem with Reactive Extensions
- Understanding Observables
- Understanding Observers
- Understanding Subjects
- Using the Async Pipe
- Using the Async Pipe with Custom Pipes
- Scaling Up Application Feature Modules
- Going Beyond the Basics
- Filtering Events
- Transforming Events
- Receiving Only Distinct Events
- Taking and Skipping Events
- Debugging with Angular 9 Ivy
- Angular Ivy Validation
- Running a Simple Unit Test
- Working with Jasmine
- Testing an Angular Component
- Working with the TestBed Class
- Testing Data Bindings
- Testing a Component with an External Template
- Testing Component Events
- Testing Output Properties
- Testing Input Properties
- Testing with Asynchronous Operations
- Testing an Angular Directive
Reviews
"The corporate training provided by Greater Insights was truly exceptional. The trainers were highly knowledgeable and engaging, making the sessions both informative and enjoyable. I gained valuable insights and practical skills that I immediately applied in my work. I highly recommend Greater Insights for their professionalism and expertise."
Rahul NiraniaSamsung 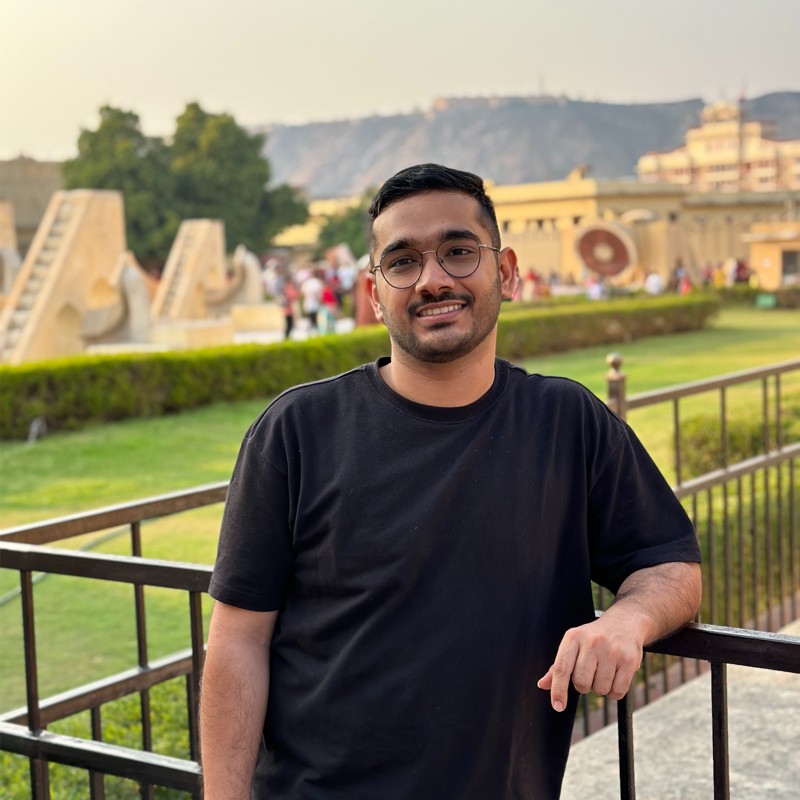
"As a participant of the corporate training program organized by Greater Insights, I was thoroughly impressed with the level of customization they offered. They took the time to understand our specific needs and tailored the training accordingly. The trainers were fantastic, and the interactive sessions fostered an environment of active learning. I am grateful for the valuable knowledge and skills I acquired."
Bhawna TiwariSamsung 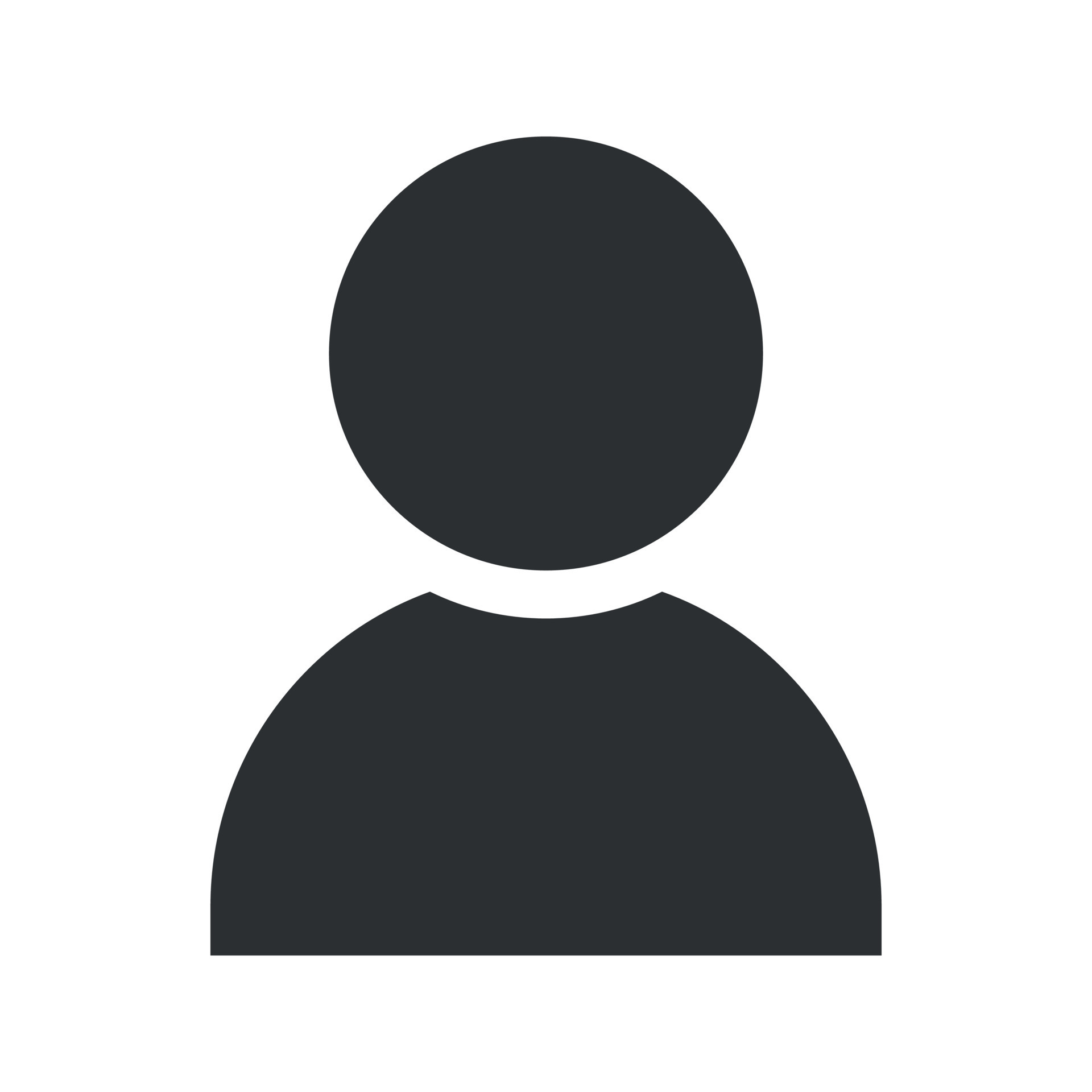
"I cannot express enough gratitude to Greater Insights for the outstanding corporate training they provided. The trainers were not only experts in their respective fields but also incredible communicators. They created a dynamic and collaborative learning environment, which allowed us to learn from one another. The training surpassed my expectations, and I would eagerly participate in any future programs they offer."
Ashutosh SinghSamsung 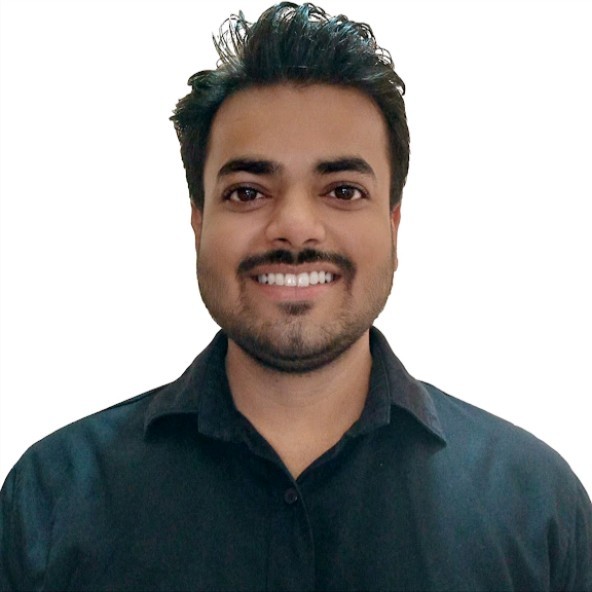
"Attending the corporate training sessions organized by Greater Insights was a game-changer for me. The trainers were not only experienced professionals but also inspiring mentors. They equipped me with practical tools and strategies that have significantly enhanced my productivity and efficiency at work. I wholeheartedly recommend Greater Insights to anyone looking to excel in their professional endeavors."
Somashekar MuniyappaInfogain 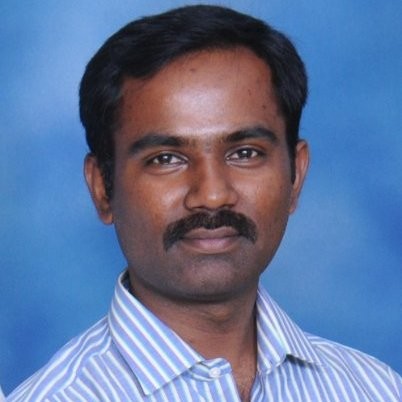
"The corporate training program offered by Greater Insights was an enlightening experience. The trainers had a deep understanding of the subject matter and were able to break down complex concepts into easily digestible information. The interactive activities and case studies made the training engaging and relevant to our day-to-day work challenges. This training has undoubtedly boosted my confidence and competence."
Amresh DiwanInfogain 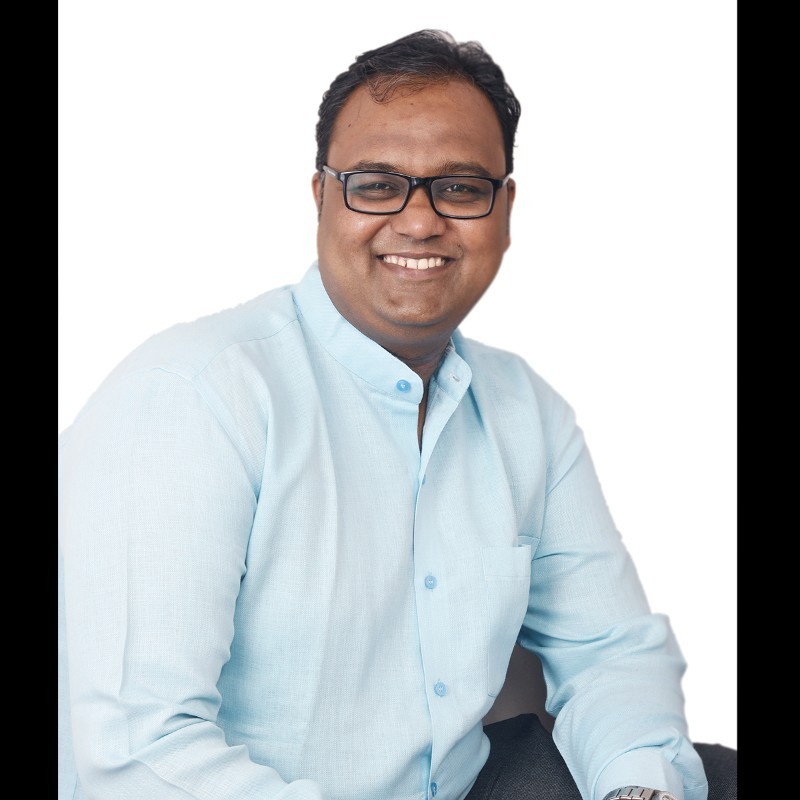
"I had the privilege of participating in a corporate training program organized by Greater Insights, and I must say it was a transformative experience. The trainers' expertise and passion for their subjects were evident in every session. The training materials provided were comprehensive and well-structured, enabling us to grasp the content effectively. I am grateful for the valuable skills I acquired, which have greatly contributed to my professional growth."
Namratha BabuTorry Harris 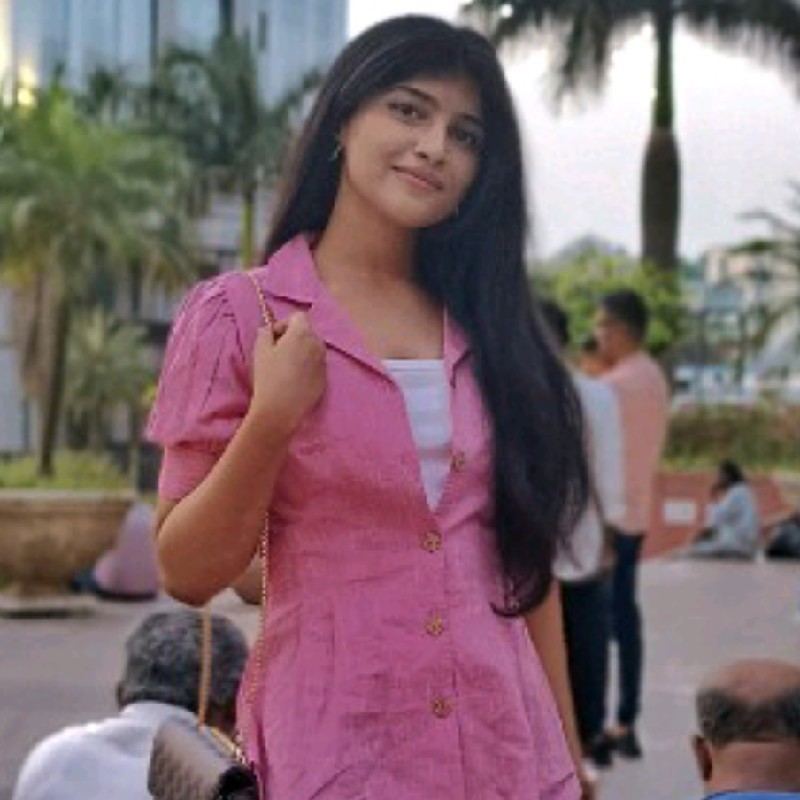
"The corporate training sessions conducted by Greater Insights were simply outstanding. The trainers went above and beyond to ensure that we understood the concepts thoroughly. The interactive nature of the sessions encouraged active participation and enhanced our learning experience. I am truly grateful for the practical strategies and techniques I learned, which have had a positive impact on my work performance."
Rahul BhashyamTorry Harris 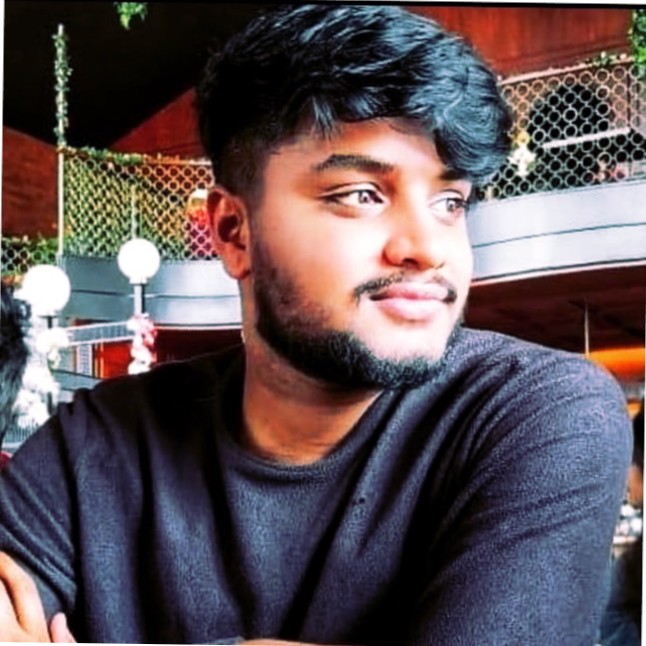
"Participating in the corporate training program organized by Greater Insights was an enlightening experience. The trainers were not only knowledgeable but also skilled at delivering the content in an engaging manner. The training materials were well-designed, and the real-life examples provided valuable insights. I am confident that the knowledge and skills I gained will significantly contribute to my professional growth."
Deepti JainTorry Harris 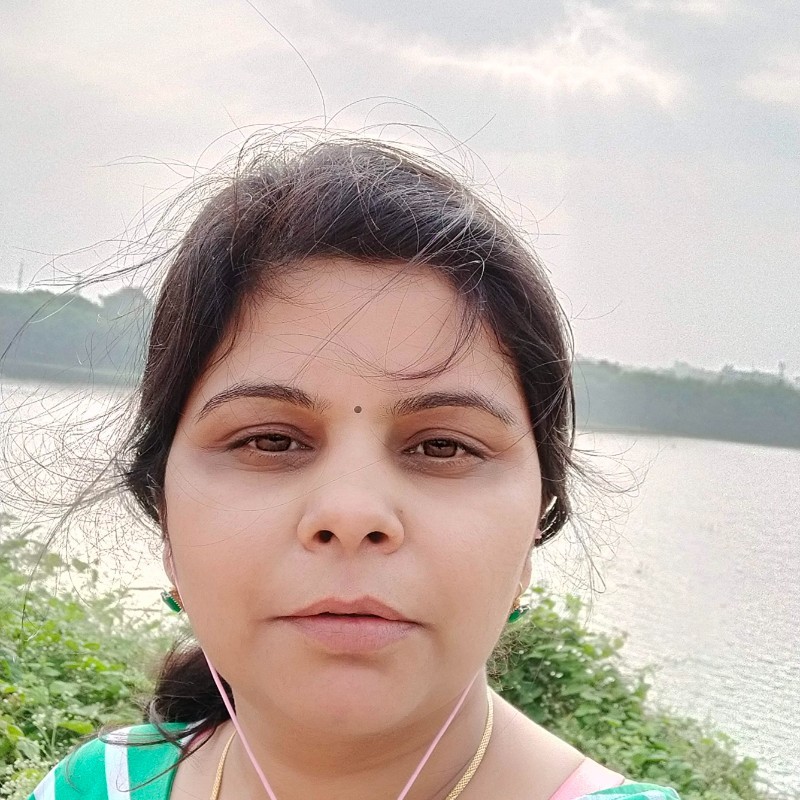
"I can confidently say that the corporate training provided by Greater Insights was top-notch. The trainers were highly experienced and had a knack for simplifying complex concepts. The training sessions were interactive and encouraged open discussions, which fostered a collaborative learning environment. The practical skills I acquired during the training have proven to be invaluable in my day-to-day work."
HARSHAL TRIVEDIL&T TS 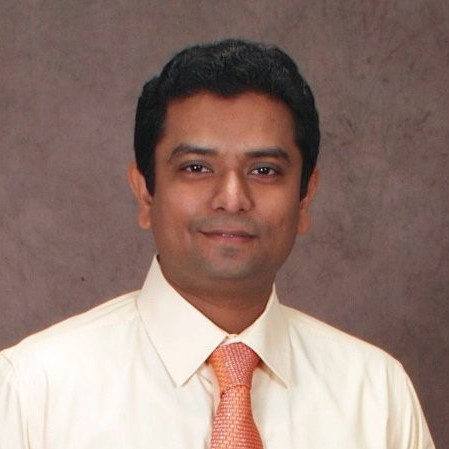
"Greater Insights exceeded my expectations with their corporate training program. The trainers were not only experts in their fields but also exceptional communicators. They effortlessly connected with the participants and ensured everyone's active involvement. The training content was comprehensive and provided a solid foundation for professional growth. I am grateful for the opportunity to learn from such seasoned professionals."
Namrata PillayL&T TS 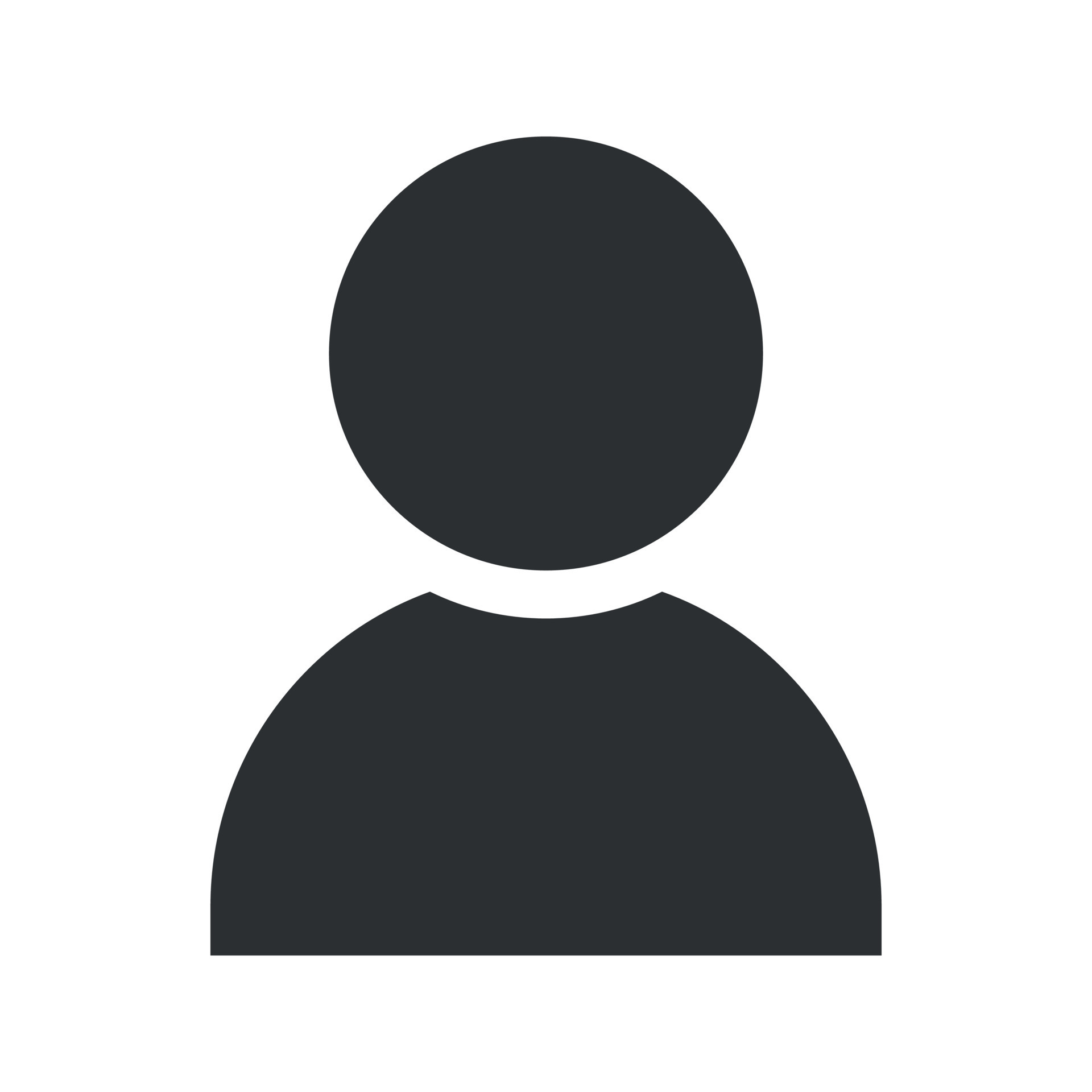
"The corporate training facilitated by Greater Insights was exceptional in every aspect. The trainers were engaging and created a positive and inclusive learning environment. The training materials were well-structured and easy to follow, making the learning process enjoyable. The practical exercises and case studies allowed me to apply the knowledge immediately, resulting in improved job performance. I highly recommend Greater Insights for their commitment to excellence."
Sandhya M SanuHoneywell 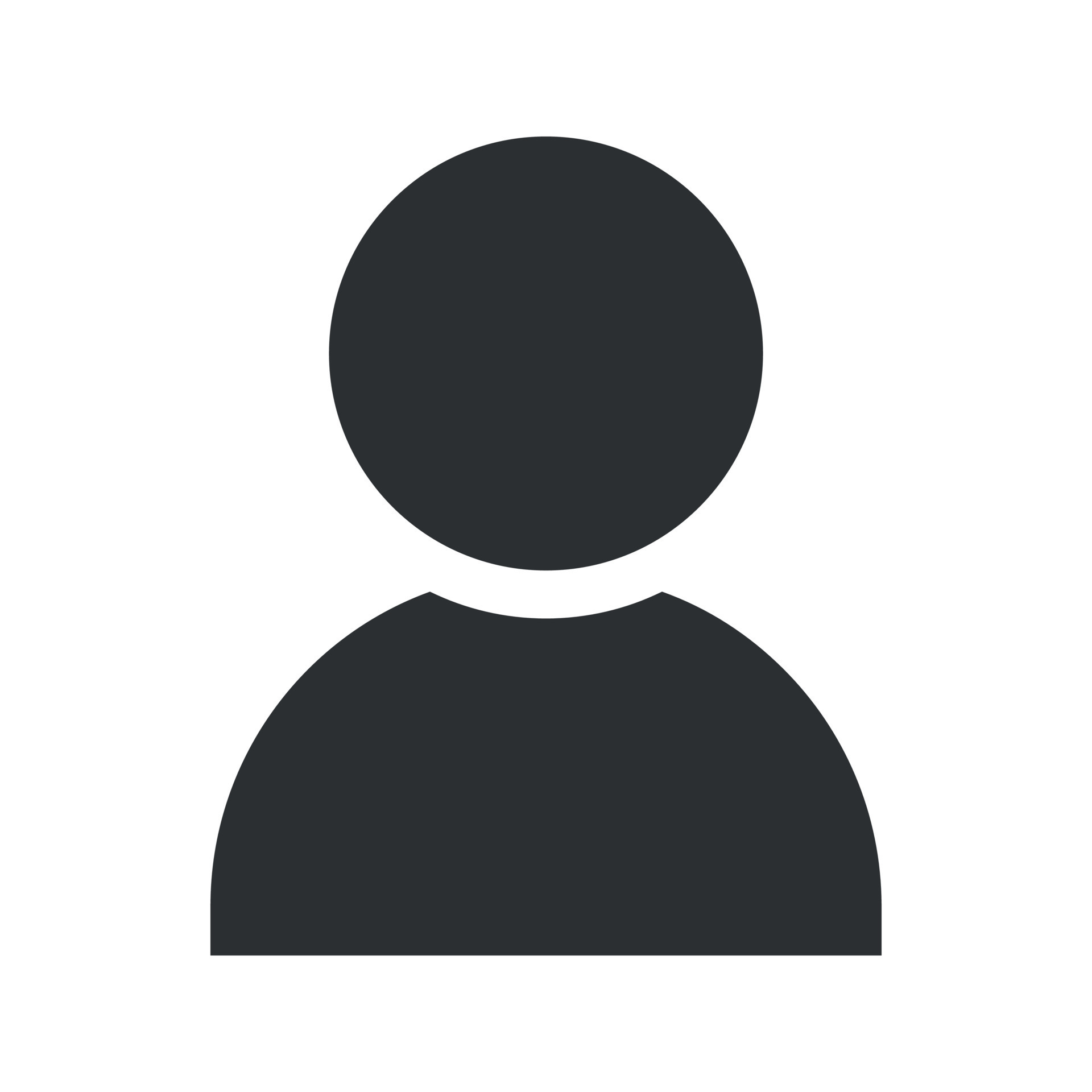
"I recently attended a corporate training program organized by Greater Insights, and I am incredibly impressed. The trainers were not only knowledgeable but also highly skilled at delivering the content in a relatable manner. The training sessions were interactive and encouraged open dialogue, which made the learning experience engaging and dynamic. The practical skills I gained have already made a significant impact on my professional growth."
Neha SahayHoneywell 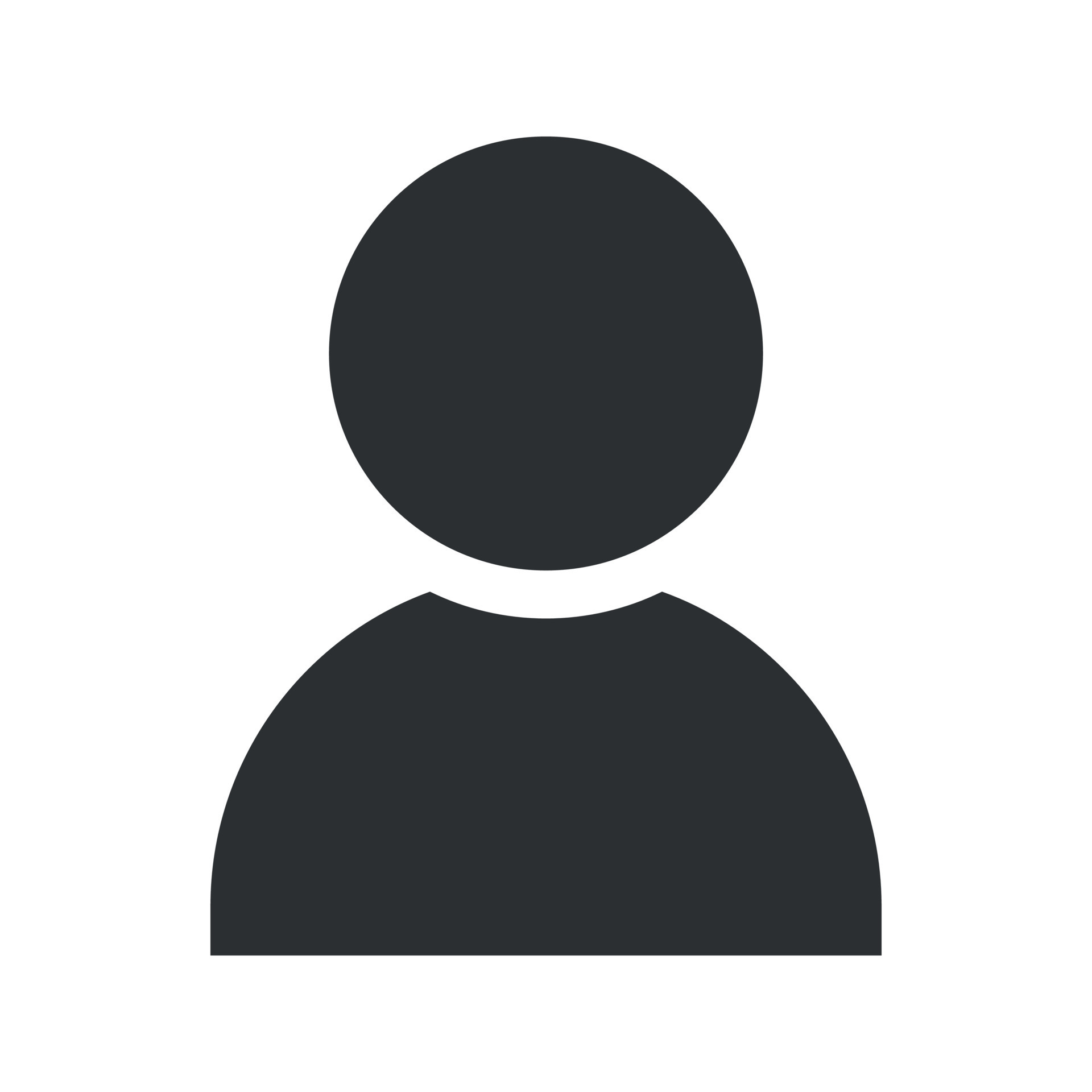
"I had the privilege of participating in a corporate training program conducted by Greater Insights, and it was truly transformative. The trainers were passionate and dedicated professionals who took the time to address our individual needs. The training sessions were interactive and encouraged active participation, allowing us to learn from one another's experiences. I am grateful for the knowledge and skills I acquired, which have undoubtedly propelled my career forward."
Abishek KalliparambilAllianz 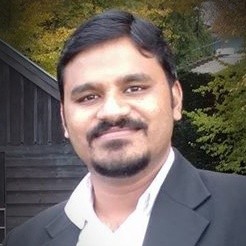
"The corporate training program offered by Greater Insights was a remarkable experience. The trainers had a wealth of knowledge and were adept at imparting it effectively. The training materials were comprehensive and provided valuable resources for further exploration. The interactive activities and practical exercises helped me internalize the concepts and apply them in real-world scenarios. This training has been instrumental in my professional development."
Kishor KumarITC Infotech 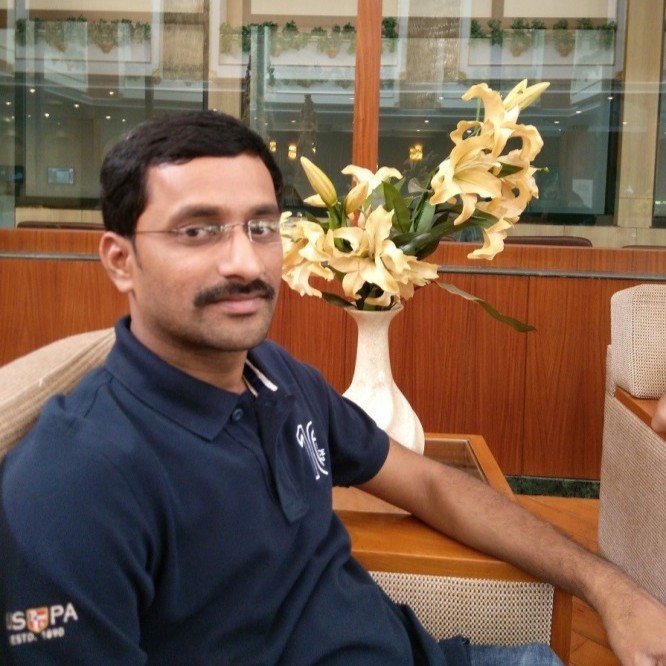
"I cannot thank Greater Insights enough for the incredible corporate training they provided. The trainers were not only subject matter experts but also skilled at creating a positive and inclusive learning environment. The training sessions were well-structured, and the trainers ensured that we understood the content thoroughly. I am grateful for the valuable insights and skills I gained, which have already made a noticeable difference in my professional life."
Anitha PrashanthIndegene 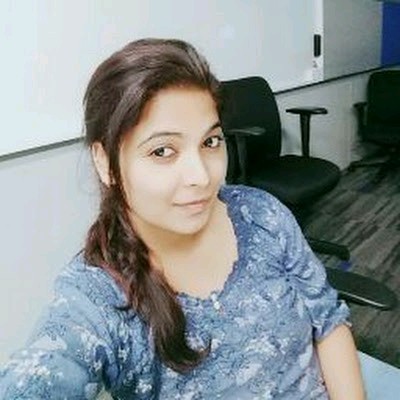